Tutorial for POPBiLL NTS Crawling
It is an example to implement SDK function ‘RequestJob’(To request a data crawling) using POPBiLL Java SDK.
- SpringMVC
- SpringBoot
- JSP
1. Add POPBiLL SDK using Maven JDK v1.6Tomcat v7STS v3.6.4
① To add POPBiLL Java SDK, input the dependency information to file "pom.xml" and update Maven.
<dependency>
<groupId>kr.co.linkhub</groupId>
<artifactId>popbill-sdk</artifactId>
<version>1.57.1</version>
</dependency>
② Add a class of e-Tax invoice as bean of Spring. Update file "servlet-context.xml" referring codes below.
Must change values of LinkID and SecretKey with the API Key issued by POPBiLL.
<?xml version="1.0" encoding="UTF-8"?>
<beans:beans xmlns="http://www.springframework.org/schema/mvc"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:beans="http://www.springframework.org/schema/beans"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:util="http://www.springframework.org/schema/util"
xsi:schemaLocation="http://www.springframework.org/schema/mvc http://www.springframework.org/schema/mvc/spring-mvc.xsd
http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context.xsd
http://www.springframework.org/schema/util http://www.springframework.org/schema/util/spring-util.xsd">
<!-- DispatcherServlet Context: defines this servlet's request-processing infrastructure -->
<!-- Enables the Spring MVC @Controller programming model -->
<annotation-driven/>
<!-- Handles HTTP GET requests for /resources/** by efficiently serving up static resources in the ${webappRoot}/resources directory -->
<resources mapping="/resources/**" location="/resources/"/>
<!-- Resolves views selected for rendering by @Controllers to .jsp resources in the /WEB-INF/views directory -->
<beans:bean class="org.springframework.web.servlet.view.InternalResourceViewResolver">
<beans:property name="prefix" value="/WEB-INF/views/"/>
<beans:property name="suffix" value=".jsp"/>
</beans:bean>
<context:component-scan base-package="com.popbill.example"/>
<util:properties id="EXAMPLE_CONFIG">
<!-- 링크아이디 -->
<beans:prop key="LinkID">TESTER</beans:prop>
<!-- 비밀키 -->
<beans:prop key="SecretKey">SwWxqU+0TErBXy/9TVjIPEnI0VTUMMSQZtJf3Ed8q3I=</beans:prop>
<!-- 연동환경 설정값 true(개발용), false(상업용) -->
<beans:prop key="IsTest">true</beans:prop>
<!-- 인증토큰 아이피 제한 기능 사용여부 권장(true) -->
<beans:prop key="IsIPRestrictOnOff">true</beans:prop>
<!-- 팝빌 API 서비스 고정 IP 사용여부, true-사용, false-미사용, 기본값(false) -->
<beans:prop key="UseStaticIP">false</beans:prop>
</util:properties>
<beans:beans>
<!-- 홈택스 전자세금계산서 Service Implementation Bean registration. -->
<beans:bean id="htTaxinvoiceService" class="com.popbill.api.hometax.HTTaxinvoiceServiceImp">
<beans:property name="linkID" value="#{EXAMPLE_CONFIG.LinkID}"/>
<beans:property name="secretKey" value="#{EXAMPLE_CONFIG.SecretKey}"/>
<beans:property name="test" value="#{EXAMPLE_CONFIG.IsTest}"/>
<beans:property name="IPRestrictOnOff" value="#{EXAMPLE_CONFIG.IsIPRestrictOnOff}"/>
<beans:property name="useStaticIP" value="#{EXAMPLE_CONFIG.UseStaticIP}"/>
</beans:bean>
</beans:beans>
</beans:beans>
2. Implement Function ‘RequestJob’
① Add @Autowired annotation and ‘requestJob’ code to create the object of service class bean.
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import com.popbill.api.HTTaxinvoiceService;
import com.popbill.api.PopbillException;
import com.popbill.api.hometax.QueryType;
@Controller
public class HTTaxinvoiceExample {
@Autowired
private HTTaxinvoiceService htTaxinvoiceService;
@RequestMapping(value = "requestJob", method = RequestMethod.GET)
public String requestJob(Model m) {
// 사업자번호
String corpNum = "1234567890";
// 전자세금계산서 유형, SELL-매출, BUY-매입, TRUSTEE-수탁
QueryType TIType = QueryType.SELL;
// 일자유형, W-작성일자, I-발행일자, S-전송일자
String DType = "S";
// 시작일자, 날짜형식(yyyyMMdd)
String SDate = "20220501";
// 종료일자, 닐짜형식(yyyyMMdd)
String EDate = "20220510";
try {
String jobID = htTaxinvoiceService.requestJob(corpNum, TIType,
DType, SDate, EDate);
m.addAttribute("Result", jobID);
} catch (PopbillException e) {
// 예외 발생 시, e.getCode() 로 오류 코드를 확인하고, e.getMessage()로 오류 메시지를 확인합니다.
System.out.println("오류 코드" + e.getCode());
System.out.println("오류 메시지" + e.getMessage());
}
return "response";
}
}
② Add a page(“src/main/webapp/WEB-INF/views/response.jsp") to output the result (code, message) of calling functions.
<%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c" %>
<%@ page pageEncoding="UTF-8" contentType="text/html; charset=UTF-8"%>
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8"/>
<title>Popbill SDK Response</title>
</head>
<body>
<p>작업아이디(JobID) : ${Result}</p>
</body>
</html>
③ Call web-browser to check the JobID returned.
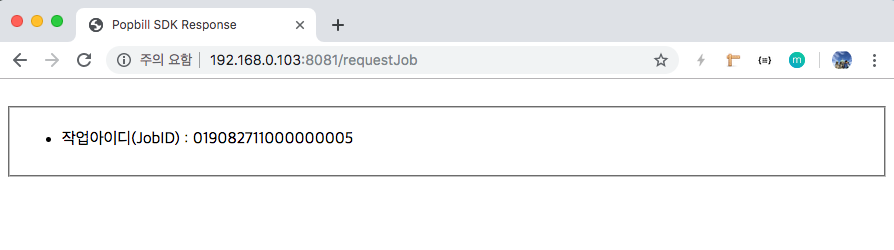
1. Add POPBiLL SDK CentOS 6.8Tomcat v7JDK v1.6
① Unzip the downloaded SDK example codes. [POPBiLL SDK Library - Java JSP Example]
② Copy 3 jar files below and Paste to library folder.

③ Create a file "$CATALINA_HOME/webapps/ROOT/common.jsp" to configure e-Tax invoice service backgrounds. Then, update the authentication information, 'LinkID' and 'SecretKey'.
Must change values of 'LinkID' and 'SecretKey' with the API Key issued by POPBiLL.
<jsp:useBean id="htTaxinvoiceService" scope="application" class="com.popbill.api.hometax.HTTaxinvoiceServiceImp" />
<%-- 링크아이디 --%>
<jsp:setProperty name="htTaxinvoiceService" property="linkID" value="TESTER" />
<%-- 비밀키, 사용자 인증에 사용되는 정보이므로 유출에 주의 --%>
<jsp:setProperty name="htTaxinvoiceService" property="secretKey" value="SwWxqU+0TErBXy/9TVjIPEnI0VTUMMSQZtJf3Ed8q3I=" />
<%-- 연동환경 설정값, 개발용(true), 상업용(false) --%>
<jsp:setProperty name="htTaxinvoiceService" property="test" value="true" />
<%-- 인증토큰 발급 IP 제한 On/Off, ture -제한기능 사용(기본값-권장), false-제한기능 미사용 --%>
<jsp:setProperty name="htTaxinvoiceService" property="IPRestrictOnOff" value="true" />
<%-- 팝빌 API 서비스 고정 IP 사용여부, true-사용, false-미사용, 기본값(false) --%>
<jsp:setProperty name="htTaxinvoiceService" property="useStaticIP" value="false"/>
2. Implement Function ‘RequestJob’
① Create file “$CATALINA_HOME/webapps/ROOT/requestJob.jsp” and Add codes like as below.
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8" />
<title>Popbill HTTaxinvoice Example</title>
</head>
<%@ include file="common.jsp" %>
<%@page import="com.popbill.api.hometax.QueryType" %>
<%@page import="com.popbill.api.PopbillException"%>
<%
/*
* 전자세금계산서 매출/매입 내역 수집을 요청합니다
* - 수집 요청후 반환받은 작업아이디(JobID)의 유효시간은 1시간 입니다.
*/
// 팝빌회원 사업자번호
String testCorpNum = "1234567890";
// SELL-매출 세금계산서, BUY-매입 세금계산서, TRUSTEE-위수탁 세금계산서
QueryType TaxinvoiceType = QueryType.SELL;
// 수집일자유형 유형, W-작성일자, I-발행일자, S-전송일자
String DType = "S";
// 시작일자, 날짜형식(yyyyMMdd)
String SDate = "20220501";
// 종료일자, 날짜형식(yyyyMMdd)
String EDate = "20220510";
String jobID = null;
try {
jobID = htTaxinvoiceService.requestJob(testCorpNum, TaxinvoiceType, DType, SDate, EDate);
} catch (PopbillException pe) {
//적절한 오류 처리를 합니다. pe.getCode() 로 오류코드를 확인하고, pe.getMessage()로 관련 오류메시지를 확인합니다.
System.out.println("오류코드 " + pe.getCode());
System.out.println("오류메시지 " + pe.getMessage());
throw pe;
}
%>
<body>
<p>Response</p>
<br/>
<fieldset>
<legend>홈택스 전자세금계산서 수집 요청</legend>
<ul>
<li>jobID (작업아이디) :<%=jobID%></li>
</ul>
</fieldset>
</body>
</html>
② Call web-browser to check the JobID returned.
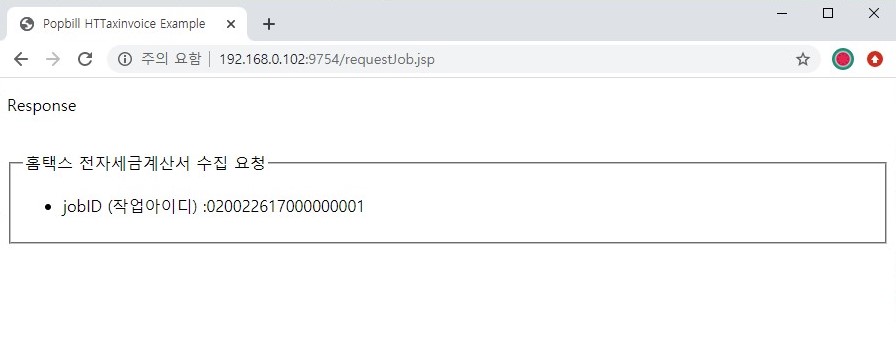
1. Add Popbill SpringBoot Starter using Gradle 
① Add dependency information to file "build.gradle" of SpringBoot project and Refresh..
※ POPBiLL SpringBoot Starter supports to SpringBoot version 1.0 and later and POPBiLL Java SDK AutoConfiguration.
dependencies {
implementation 'kr.co.linkhub:popbill-spring-boot-starter:1.4.0'
}
② Add the code below to file “appllication.yml” for setup SDK configuration.
Must change values of ‘LinkID’ and ‘SecretKey’ with the API Key issued by POPBiLL.
popbill:
#링크아이디
linkId: TESTER
#비밀키
secretKey: SwWxqU+0TErBXy/9TVjIPEnI0VTUMMSQZtJf3Ed8q3I=
#연동환경 설정값 true(개발용), false(상업용)
isTest: true
#인증토큰 아이피 제한 기능 사용여부 true(사용-권장), false(미사용)
isIpRestrictOnOff: true
#팝빌 API 서비스 고정 IP 사용여부 true(사용), false(미사용)
useStaticIp: false
#로컬시스템 시간 사용여부 true(사용-권장), false(미사용)
useLocalTimeYn: true
2. 홈택스 전자세금계산서 수집 요청(RequestJob) 기능 구현
① @Autowired 어노테이션을 이용하여 생성된 서비스 클래스 빈 객체를 주입 받은 후 requestJob 함수 코드를 추가합니다.
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import com.popbill.api.HTTaxinvoiceService;
import com.popbill.api.PopbillException;
import com.popbill.api.hometax.QueryType;
@Controller
public class HTTaxinvoiceServiceController {
@Autowired
private HTTaxinvoiceService htTaxinvoiceService;
@RequestMapping(value = "requestJob", method = RequestMethod.GET)
public String requestJob(Model m) {
// 사업자번호
String corpNum = "1234567890";
// 전자세금계산서 유형, SELL-매출, BUY-매입, TRUSTEE-수탁
QueryType TIType = QueryType.SELL;
// 일자유형, W-작성일자, I-발행일자, S-전송일자
String DType = "S";
// 시작일자, 날짜형식(yyyyMMdd)
String SDate = "20220101";
// 종료일자, 닐짜형식(yyyyMMdd)
String EDate = "20220130";
try {
String jobID = htTaxinvoiceService.requestJob(corpNum, TIType,
DType, SDate, EDate);
m.addAttribute("Result", jobID);
} catch (PopbillException e) {
// 예외 발생 시, e.getCode() 로 오류 코드를 확인하고, e.getMessage()로 오류 메시지를 확인합니다.
System.out.println("오류 코드" + e.getCode());
System.out.println("오류 메시지" + e.getMessage());
}
return "response";
}
}
② 함수 호출결과 코드와 메시지를 매핑하는 "src/main/resources/templates/response.html" 페이지를 추가합니다.
<html xmlns:th="http://www.thymeleaf.org"">
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8"/>
<title>Popbill SDK Response</title>
</head>
<body>
<ul>
<li>작업아이디(JobID) : <span th:text="${Result}"></span></li>
</ul>
</body>
</html>
③ 웹브라우저 페이지를 호출하여 함수호출 결과로 반환되는 작업아이디(JobID)를 확인합니다.
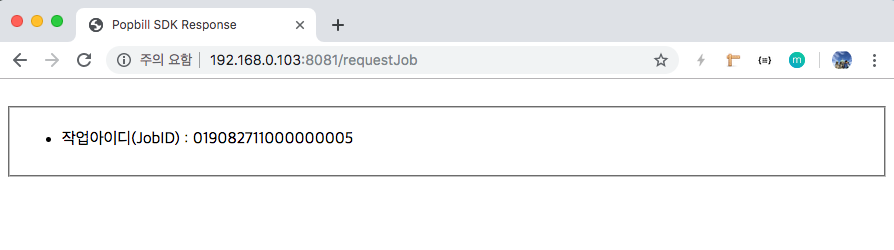
Business Contact
For more help with POPBiLL, try these resources :
T. +82 70-7998-7117E. global@linkhubcorp.com