POPBiLL Event Webhook
POPBiLL Event Webhook is an incoming webhook service to receive sales/purchase invoices crawled from NTS, every hour on the hour. Crawled invoices are delivered to registered call-back URL as real-time HTTP event messages and it make the better business efficiency to save the manual effort.
Process of event webhook
1) Occur a webhook event : POPBiLL check a user's call-back URL.
2) HTTP Request : POPBiLL send a webhook message as a HTTP POST Request type to this call-back URL.
3) Check user's request : User check a JSON Object on Body of HTTP POST Request.
4) HTTP Response
In case of 'success', the value of OK(String Type) or {result : 'OK'}(JSON Object) is going to be returned as a HTTP Response.
In case of 'fail' caused by HTTP network error (e.g. Read timeout, Gateway timeout, SSL error etc.), the webhook is going to be retried twice at 5-minute intervals.
5) Check the response result
POPBiLL determine whether the webhook is success or fail, depending on the response result. When partner's server error is expected by monitoring system, POPBiLL give a notice directly to partner.
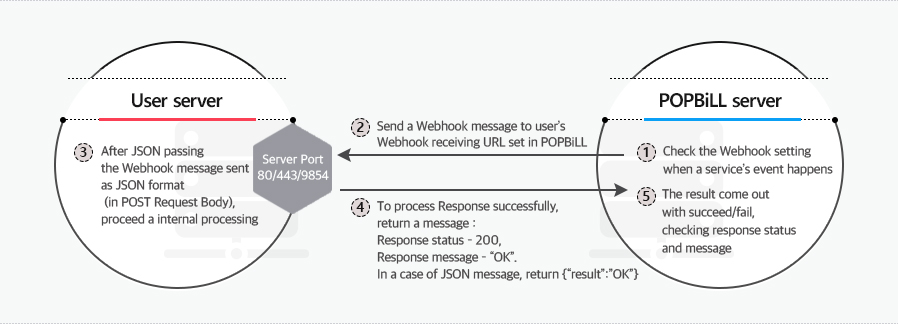
Webhook Type and Configuration
POPBiLL supports 2 webhook types and please refer the table below for configuration method.
- | Common Webhook | Individual Webhook |
---|---|---|
Subject |
All webhook of partner's user
are delivered to an identical(common) URL |
Each webhook of partner's user
is delivered to designated individual URL |
How to configure |
POPBiLL takes in charge of configuration
> User sends a call-back URL to POPBiLL by email (global@linkhubcorp.com) |
1. Login POPBiLL website
2. Access menu [ 홈택스연동(NTS Crawling) > Webhook 관리(Webhook Configuration) > Webhook 등록(Webhook Registration) ] 3. Set Webhook 유형(Webhook Type) as REST 4. Set 콜백 URL(Call-back URL) 5. Set Webhook 인증(Webhook authentication) as '미사용'(Not in use) |
※ You can use 80, 443 or 9854 port as a call-back URL. If you need to use another port, please contact technical support center.
Example codes of receiving Webhook - SpringMVC
1) Add the 'gson' dependency information to handle event message in JSON format and update Maven.
<dependency>
<groupId>com.google.code.gson</groupId>
<artifactId>gson</artifactId>
<version>2.3</version>
</dependency>
2) Add a functionality to handle 'POST Request Body'.
ㆍIt's an example code to set an address of Webhook receiving URL as 'http(s)://Your URL/pbconnect'.
import java.io.BufferedReader;
import javax.servlet.http.HttpServletRequest;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.bind.annotation.ResponseBody;
import com.google.gson.JsonObject;
import com.google.gson.JsonParser;
@Controller
public class HomeController {
@ResponseBody
@RequestMapping(value = "pbconnect", method = RequestMethod.POST)
public String webhook(HttpServletRequest request){
StringBuffer strBuffer = new StringBuffer();
String line = null;
try {
BufferedReader reader = request.getReader();
while ((line = reader.readLine()) != null) {
strBuffer.append(line);
}
} catch (Exception e) {
System.out.println("Error reading JSON string: " + e.toString());
// 오류정보를 Return 처리. 팝빌 Webhook 실행내역에서 확인가능
return e.toString();
}
// Reqeust Body 출력
System.out.println(strBuffer.toString());
// Request Body JSON 처리.
// 자세한 Request Body 항목은 하단의 [Webhook 이벤트 메시지 구성] 참조
JsonParser parser = new JsonParser();
JsonObject jsonObject = (JsonObject)parser.parse(strBuffer.toString());
System.out.println("eventType : " + jsonObject.get("eventType"));
System.out.println("eventDT : " + jsonObject.get("eventDT"));
// Webhook 수신을 성공으로 처리하기 위해 JSON String 구성
return "{'result':'OK'}";
}
}
Schema of Webhook event messages
1) Header of event message
List name | Definition | Examples |
---|---|---|
Accept | Media Server Type | text/plain, */* |
User-Agent | Asked Server Information | Popbill Webhook executor (TAXINVOICE.STATE) |
Pb-Webhook-Type | Event Type | TAXINVOICE.STATE |
Pb-Webhook-MID | Event Verification Value | 016120000002-1777d55c2c41492ab06826d |
Pb-Webhook-Corpnum | Business Registration Number | 6798700433 |
Content-Type | Body Type of Webhook Message | application/json |
Host | Host Information | docs.popbill.com |
Connection | Webhook Configuration | keep-alive |
Content-Length | Body Length of Webhook Message | 243 |
Authorization | Webhook Authentication – using BASIC | Basic VEVTVDoxMjM= |
X-Api-Key | Webhook Authentication – using API KEY | TEST |
2) Body of event message
List name | Type | Length | Notes |
---|---|---|---|
writeDate | String | 8 |
Date of preparation
└ Format : yyyyMMdd |
ntsconfirmNum | String | 24 | NTS confirm number |
issueDT | String | 14 |
Date and Time of issuance
└ Format : yyyyMMdd |
invoiceType | Number | 3 |
Type of invoice
└ 101 : e-Tax invoice, 102 : Zero-rate invoice └ 103 : Consigned invoice, 104 : e-Tax invoice on import └ 105 : Consigned zero-rated invoice └ 201 : Revised invoice, 202 : Revised zero-rated invoice └ 203 : Revised consigned invoice, 204 : Revised invoice on import └ 205 : Revised consigned zero-rated invoice └ 301 : e-Invoice, 303 : Consigned e-Invoice, 304 : e-Invoice on import └ 401 : Revised e-Invoice, 403 : Revised consigned e-Invoice └ 404 : Reviced e-Invoice on import |
taxType | String | 2 |
Taxation Type
└ 과세 = Taxable, 영세 = Zero-rate, 면세 = Exempted |
taxTotal | String | 18 | Sum of tax amount |
supplyCostTotal | String | 18 | Sum of supply values |
totalAmount | String | 18 |
Total Amount
└ sum of taxTotal and supplyCostTotal |
purposeType | String | 2 |
Type of the total amount
└ Valid Value : 영수 / 청구 / 없음 └ 영수 = Amount paid, 청구 = Amount due, 없음 = None |
serialNum | String | 30 | One of e-Tax invoice's items to manage document – 'Serial number' |
cash | String | 18 | Payment Method – 'cash' |
chkBill | String | 18 | Payment Method – 'check' |
credit | String | 18 | Payment Method – 'credit' |
note | String | 18 | Payment Method – ‘note' |
remark1 | String | 150 | 1st Remark |
remark2 | String | 150 | 2nd Remark |
remark3 | String | 150 | 3rd Remark |
invoicerCorpNum | String | 10 | [Seller] Business Registration Number |
invoicerMgtKey | String | 24 | [Seller] Invoice ID |
invoicerTaxRegID | String | 4 |
[Seller] Identification Number for minor place of business
└ 4 digits number |
invoicerCorpName | String | 200 | [Seller] Name of Company |
invoicerCEOName | String | 100 | [Seller] Name of Representative |
invoicerAddr | String | 300 | [Seller] Company Address |
invoicerBizType | String | 100 | [Seller] Business Type |
invoicerBizClass | String | 100 | [Seller] Business Item |
invoicerContactName | String | 100 | [Seller] Name of the person in charge |
invoicerDeptName | String | 100 | [Seller] Department of the person in charge |
invoicerTEL | String | 20 | [Seller] Telephone of the person in charge |
invoicerEmail | String | 100 | [Seller] Email address of the person in charge |
invoiceeCorpNum | String | 10 |
[Buyer] Business Registration Number
└ It must be synchronized with invoiceeType └ Company: Business registration number (10 digits except '-') └ Individual: Resident registration number (13 digits except '-') └ Foreigner: "9999999999999"(13 digits except '-') |
invoiceeType | String | 3 |
Buyer Type
└ Valid Value : 사업자 / 개인 / 외국인 └ 사업자 = company, 개인 = individual, 외국인 = foreigner |
invoiceeMgtKey | String | 24 | It's only requires to 'requested e-Tax invoice' |
invoiceeTaxRegID | String | 4 |
[Buyer] Identification Number for minor place of business
└ 4 digits number |
invoiceeCorpName | String | 200 | [Buyer] Name of Company |
invoiceeCEOName | String | 100 | [Buyer] Name of Representative |
invoiceeAddr | String | 300 | [Buyer] Company Address |
invoiceeBizType | String | 100 | [Buyer] Business Type |
invoiceeBizClass | String | 100 | [Buyer] Business Item |
invoiceeContactName1 | String | 100 | [Buyer] Name of the person in charge |
invoiceeDeptName1 | String | 100 | [Buyer] Department of the person in charge |
invoiceeTEL1 | String | 20 | [Buyer] Telephone of the person in charge |
invoiceeEmail1 | String | 100 | [Buyer] Email address of the person in charge |
invoiceeContactName2 | String | 100 | [Buyer] Name of the 2nd person in charge |
invoiceeDeptName2 | String | 100 | [Buyer] Department of the 2nd person in charge |
invoiceeTEL2 | String | 20 | [Buyer] Telephone of the 2nd person in charge |
invoiceeEmail2 | String | 100 | [Buyer] Email of the 2nd person in charge |
trusteeCorpNum | String | 10 | [Consignee] Business Registration Number |
trusteeMgtKey | String | 24 | [Consignee] Invoice ID |
trusteeTaxRegID | String | 4 |
[Consignee] Identification Number for minor place of business
└ 4 digits number |
trusteeCorpName | String | 200 | [Consignee] Name of Company |
trusteeCEOName | String | 100 | [Consignee] Name of Representative |
trusteeAddr | String | 300 | [Consignee] Company Address |
trusteeBizType | String | 100 | [Consignee] Business Type |
trusteeBizClass | String | 100 | [Consignee] Business Item |
trusteeContactName | String | 100 | [Consignee] Name of the person in charge |
trusteeDeptName | String | 100 | [Consignee] Department of the person in charge |
trusteeTEL | String | 20 | [Consignee] Telephone of the person in charge |
trusteeEmail | String | 100 | [Consignee] Email address of the person in charge |
modifyCode | Number | 1 |
Modification Code
└ Valid Value : 1 / 2 / 3 / 4 / 5 / 6 └ 1 = Correction of errors, 2 = Change in supply values, 3 = Return └ 4 = Cancellation of contract, 5 = Post opening of local letter of credit └ 6 = Duplicated issuance |
orgNTSConfirmNum | String | 24 | NTS Confirm Number of the Original |
detailList | Array | 99 | [List] Detailed list of items (Maximum : 99) |
┖ serialNum | Number | 2 | Serial Number |
┖ purchaseDT | String | 8 |
Date of preparation
└ Format : yyyyMMdd |
┖ itemName | String | 100 | Item name |
┖ spec | String | 60 | Specification |
┖ qty | String | 12 |
Quantity
└ Available to two decimal places └ Minus(Negative) amount is available |
┖ unitCost | String | 18 |
Unit price
└ Available to two decimal places └ Minus(Negative) amount is available |
┖ supplyCost | String | 18 |
Unit price
└ Minus(Negative) amount is available |
┖ tax | String | 18 |
Tax amount
└ Minus(Negative) amount is available |
┖ remark | String | 100 | Remark |
Schema of Webhook response messages
To make a success of Webhook event execution, you need to setup the body of response message is going to return a one of them below.
Definition | String Type | JSON Type |
---|---|---|
Body Message | "OK" | { "result":"OK" } |
Checking the execution details about Webhook event and retry
- Sign in POPBiLL website
- Access menu [홈택스연동] (NTS Crawling) on the top > [Webhook 관리] (Webhook management) on left side menu > [전자세금계산서 실행내역] (execution details of e-Tax invoice crawling from NTS)
- Retry after checking the detailed message of it
Business Contact
For more help with POPBiLL, try these resources :
T. +82 70-7998-7117E. global@linkhubcorp.com