POPBiLL Event Webhook
The webhook is a Webhook incoming service to check real-time status information of e-Cashbill issued through POPBiLL. It's not required to call the function to check the e-Cashbill's status as the former(API method). Using designated call-back URL, you can receive real-time HTTP event messages and check real-time status information of e-Cashbill more easily.
Process of webhook service
1) Occur a webhook event : POPBiLL check a partner's call-back URL.
2) HTTP Request : POPBiLL send a webhook message as a HTTP POST Request type to this call-back URL.
3) Check the partner's request : Partner check a value transferred as JSON type on Body of HTTP POST Request.
4) HTTP Response
In case of 'success', the value of OK(String Type) or {result : 'OK'}(JSON Object) is going to be returned as a HTTP Response.
In case of 'fail' caused by HTTP communication error (e.g. Read timeout, Gateway timeout, SSL error etc.), the webhook is going to be retried three times at 5-minute intervals.
5) Check the response result
POPBiLL determine whether the webhook is success or fail depending on the response result and give a notice directly to partner when we notice partner's server error monitoring about failed cases regularly.
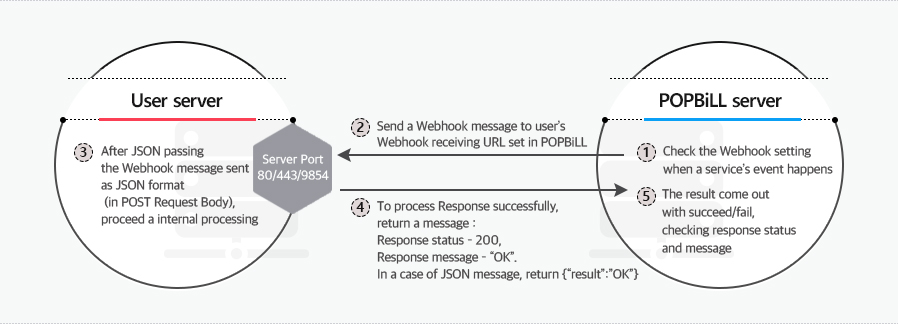
Webhook event list provided real-time
- Notification of issuance/issuance cancellation
- Notification of filing start
- Notification of filing result to NTS (Succeed/Failed)
Webhook type and how to set
POPBiLL provides two methods of webhook and you can check how to set in table below.
- | Common Webhook | Individual Webhook |
---|---|---|
Type | All webhook of partner's user
are delivered to an identical URL |
Each webhook of partner's user
is delivered to designated individual URL |
How to set |
Set by POPBiLL
> Service provider(Partner) sends call-back URL by e-mail (global@linkhubcorp.com) |
1. Login POPBiLL website
2. Access menu [ 현금영수증(e-Cashbill) > Webhook 관리(Webhook Management) > Webhook 등록(Webhook Registration) ] 3. Set Webhook 유형(Webhook type) as REST 4. Enter 콜백 URL(Call-back URL) as designated URL 5. Set Webhook 인증(Webhook authentication) as '미사용'(Not in use) |
※ You can use 80, 443 or 9854 port as a call-back URL. If you have to use another port or set same webhook call-back URL to many partner's users, contact technical support center.
Example codes of receiving Webhook - SpringMVC
1) Add the 'gson' dependency information to handle event message in JSON format and update Maven.
<dependency>
<groupId>com.google.code.gson</groupId>
<artifactId>gson</artifactId>
<version>2.3</version>
</dependency>
2) Add a functionality to handle 'POST Request Body'.
ㆍIt's an example code to set an address of Webhook receiving URL as 'http(s)://Your URL/pbconnect'.
import java.io.BufferedReader;
import javax.servlet.http.HttpServletRequest;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.bind.annotation.ResponseBody;
import com.google.gson.JsonObject;
import com.google.gson.JsonParser;
@Controller
public class HomeController {
@ResponseBody
@RequestMapping(value = "pbconnect", method = RequestMethod.POST)
public String webhook(HttpServletRequest request){
StringBuffer strBuffer = new StringBuffer();
String line = null;
try {
BufferedReader reader = request.getReader();
while ((line = reader.readLine()) != null) {
strBuffer.append(line);
}
} catch (Exception e) {
System.out.println("Error reading JSON string: " + e.toString());
// 오류정보를 Return 처리. 팝빌 Webhook 실행내역에서 확인가능
return e.toString();
}
// Reqeust Body 출력
System.out.println(strBuffer.toString());
// Request Body JSON 처리.
// 자세한 Request Body 항목은 하단의 [Webhook 메시지 구성] 참조
JsonParser parser = new JsonParser();
JsonObject jsonObject = (JsonObject)parser.parse(strBuffer.toString());
System.out.println("eventType : " + jsonObject.get("eventType"));
System.out.println("eventDT : " + jsonObject.get("eventDT"));
// Webhook 수신을 성공으로 처리하기 위해 JSON String 구성
return "{'result':'OK'}";
}
}
Configuration of connect event messages
1) Header configuration of event message
Header Fields | Definition | Examples |
---|---|---|
Pb-Webhook-Type | Service Type | CASHBILL.STATE |
Pb-Webhook-EventType | Event Type | Issue |
Pb-Webhook-MID | Event Verification Value | 016120000002-1777d55c2c41492ab06826d |
Pb-Webhook-Corpnum | Business Registration Number | 6798700433 |
Content-Type | Body Type of Webhook Message | application/json |
Authorization | Webhook Authentication - using BASIC | Basic VEVTVDoxMjM= |
X-Api-Key | Webhook Authentication - using API KEY | TESTAPIKEY |
2) Body configuration of event message
List name | Definition | Type | Length | Nullable | Notes |
---|---|---|---|---|---|
corpNum | Business Registration Number | String | 10 | - | [DEPRECATED] Use franchiseCorpNum instead. |
franchiseCorpNum | Business Registration Number | String | 10 | - | of Seller |
itemKey | POPBiLL Cashbill ID | String | 18 | - | assigned by POPBiLL automatically |
tradeDate | Date of trading | String | 8 | - | Format : yyyyMMdd |
confirmNum | NTS Confirm Number | String | 9 | - | |
ntssendDT | Date and Time of NTS Filing | String | 14 | O | Format : yyyyMMddHHmmss |
ntsresultDT | Date and Time of receiving filing result | String | 14 | O | Format : yyyyMMddHHmmss |
ntsresultCode | Result Code of NTS filing | String | 8 | O | [Reference] Result code of filing to NTS |
stateCode | Status Code | Number | 3 | - | |
stateDT | Date and Time of Status Change | String | 14 | - | Format : yyyyMMddHHmmss |
issueDT | Date and Time of issuance | String | 14 | - | Format : yyyyMMddHHmmss |
mgtKey | Cashbill ID | String | 24 | O | assigned by seller |
eventDT | Date and Time of Event executed | String | 14 | - | Format : yyyyMMddHHmmss |
eventType | Event type | String | 30 | - |
Valid Value : Issue / NTS
└ Issue : Issued └ NTS : Processing, Succeed, Failed |
interOPYN | Y/N for API transaction | Boolean | - | - |
Valid Value : true / false
└ true : issued by API transaction └ false : issued through POPBiLL website |
Schema of Webhook response messages
To make a success of Webhook event execution, you need to setup the body of response message is going to return a one of them below.
Definition | String Type | JSON Type |
---|---|---|
Body message | "OK" |
{
"result":"OK" } |
The request body example of webhook messages
Event Type | HTTP Request Body | Explanations |
---|---|---|
Issue | { "corpNum": "1234567890", "franchiseCorpNum": "1234567890", "itemKey": "022122116193800001", "tradeDate": "20221221", "confirmNum": "TB0001147", "stateCode": 300, "stateDT": "20221221161938", "issueDT": "20221221161938", "mgtKey": "2021121-002", "eventDT": "20221221161938", "eventType": "Issue", "interOPYN": true } |
- It’s identified by Seller Cashbill ID (variable: MgtKey) assigned automatically when e-Cashbill is issued/saved - Executed event return "Issue" as a value of action field - tateCode of issuance completion: Return 300 |
NTS | { "corpNum": "1234567890", "franchiseCorpNum": "1234567890", "itemKey": "022122116191200001", "tradeDate": "20221221", "confirmNum": "TB0001146", "ntssendDT": "20221222000000", "ntsresultDT": "20221222100033", "ntsresultCode": "0000", "stateCode": 304, "stateDT": "20221221161912", "issueDT": "20221221161912", "mgtKey": "20221221-001", "eventDT": "20221222100033", "eventType": "NTS", "interOPYN": true } |
- Executed event return “NTS" as a value of action field - stateCode Succeed/Failed : Return 304/305 |
※ A value of e-Cashbill state(parameter: stateCode) is not updated to smaller value.
The example of e-Cashbill Webhook event messages
- Event messages are sent to Webhook call-back URL set in POPBiLL Webhook server as HTTP Post Method and Body is consist of JSON format. The video below is the example of event messages to demonstrate how to implement process of e-Cashbill issuance/ cancel the issuance.
Checking the execution details about Webhook event and retry
- Sign in POPBiLL website
- Click menu [현금영수증](e-Cashbill) on the top > [Webhook 관리](Webhook management) on left side menu > [실행내역] (execution details)
- Retry after checking the detailed message of it
Business Contact
For more help with POPBiLL, try these resources :
T. +82 70-7998-7117E. global@linkhubcorp.com