Tutorial for POPBiLL e-Statement
It is an example to implement SDK function 'RegistIssue'(To issue an e-Statement) using POPBiLL Java SDK.
- SpringMVC
- SpringBoot
- JSP
1. Add POPBiLL SDK using Maven JDK v1.6Tomcat v7STS v3.6.4
① To add POPBiLL Java SDK, input the dependency information to file "pom.xml" and update Maven.
<dependency>
<groupId>kr.co.linkhub</groupId>
<artifactId>popbill-sdk</artifactId>
<version>1.57.1</version>
</dependency>
② Add a class of e-Tax invoice as bean of Spring. Update file "servlet-context.xml" referring codes below.
Must change values of LinkID and SecretKey with the API Key issued by POPBiLL.
<?xml version="1.0" encoding="UTF-8"?>
<beans:beans xmlns="http://www.springframework.org/schema/mvc"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:beans="http://www.springframework.org/schema/beans"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:util="http://www.springframework.org/schema/util"
xsi:schemaLocation="http://www.springframework.org/schema/mvc http://www.springframework.org/schema/mvc/spring-mvc.xsd
http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context.xsd
http://www.springframework.org/schema/util http://www.springframework.org/schema/util/spring-util.xsd">
<!-- DispatcherServlet Context: defines this servlet's request-processing infrastructure -->
<!-- Enables the Spring MVC @Controller programming model -->
<annotation-driven/>
<!-- Handles HTTP GET requests for /resources/** by efficiently serving up static resources in the ${webappRoot}/resources directory -->
<resources mapping="/resources/**" location="/resources/"/>
<!-- Resolves views selected for rendering by @Controllers to .jsp resources in the /WEB-INF/views directory -->
<beans:bean class="org.springframework.web.servlet.view.InternalResourceViewResolver">
<beans:property name="prefix" value="/WEB-INF/views/"/>
<beans:property name="suffix" value=".jsp"/>
</beans:bean>
<context:component-scan base-package="com.popbill.example"/>
<util:properties id="EXAMPLE_CONFIG">
<!-- 링크아이디 -->
<beans:prop key="LinkID">TESTER</beans:prop>
<!-- 비밀키 -->
<beans:prop key="SecretKey">SwWxqU+0TErBXy/9TVjIPEnI0VTUMMSQZtJf3Ed8q3I=</beans:prop>
<!-- 연동환경 설정값 true(개발용), false(상업용) -->
<beans:prop key="IsTest">true</beans:prop>
<!-- 인증토큰 아이피 제한 기능 사용여부 권장(true) -->
<beans:prop key="IsIPRestrictOnOff">true</beans:prop>
<!-- 팝빌 API 서비스 고정 IP 사용여부, true-사용, false-미사용, 기본값(false) -->
<beans:prop key="UseStaticIP">false</beans:prop>
</util:properties>
<beans:beans>
<!-- 전자명세서 Service Implementation Bean registration. -->
<beans:bean id="statementService" class="com.popbill.api.statement.StatementServiceImp">
<beans:property name="linkID" value="#{EXAMPLE_CONFIG.LinkID}"/>
<beans:property name="secretKey" value="#{EXAMPLE_CONFIG.SecretKey}"/>
<beans:property name="test" value="#{EXAMPLE_CONFIG.IsTest}"/>
<beans:property name="IPRestrictOnOff" value="#{EXAMPLE_CONFIG.IsIPRestrictOnOff}"/>
<beans:property name="useStaticIP" value="#{EXAMPLE_CONFIG.UseStaticIP}"/>
</beans:bean>
</beans:beans>
</beans:beans>
2. Implement Function 'RegistIssue'
① Add @Autowired annotation and registIssue code to create the object of service class bean.
import java.util.ArrayList;
import java.util.HashMap;
import java.util.Map;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import com.popbill.api.PopbillException;
import com.popbill.api.Response;
import com.popbill.api.StatementService;
import com.popbill.api.statement.Statement;
import com.popbill.api.statement.StatementDetail;
@Controller
public class StatementServiceExample {
@Autowired
private StatementService statementService;
@RequestMapping(value = "registIssue", method = RequestMethod.GET)
public String registIssue(Model m) {
// 발행자 사업자번호
String corpNum = "1234567890";
// 팝빌회원 아이디
String testUserID = "testkorea";
// 발행안내 메일 제목, 미기재시 기본 양식으로 전송
String emailSubject = "메일제목 테스트";
// 메모
String Memo = "전자명세서 즉시발행 메모";
// 전자명세서 정보 객체
Statement statement = new Statement();
// [필수] 작성일자, 형태 yyyyMMdd
statement.setWriteDate("20191004");
// [필수] {영수, 청구} 중 기재
statement.setPurposeType("영수");
// [필수] {과세, 영세, 면세} 중 기재
statement.setTaxType("과세");
// 맞춤양식코드, 미기재시 기본양식으로 처리
statement.setFormCode("");
// [필수] 명세서 코드, [121 - 거래명세서], [122 - 청구서], [123 - 견적서], [124 - 발주서], [125 - 입금표], [126 - 영수증]
statement.setItemCode((short) 121);
// [필수] 문서번호, 최대 24자리 영문, 숫자, '-', '_'로 구성
statement.setMgtKey("20190827-001");
/*********************************************************************
* 발신자 정보
*********************************************************************/
// [필수] 발신자 사업자번호
statement.setSenderCorpNum(corpNum);
// [필수] 발신자 상호
statement.setSenderCorpName("발신자 상호");
// 발신자 주소
statement.setSenderAddr("발신자 주소");
// [필수] 발신자 대표자 성명
statement.setSenderCEOName("발신자 대표자 성명");
// 발신자 종사업장 식별번호, 숫자 4자리, 필요시 기재
statement.setSenderTaxRegID("");
// 발신자 종목
statement.setSenderBizClass("업종");
// 발신자 업태
statement.setSenderBizType("업태");
// 발신자 담당자명
statement.setSenderContactName("발신자 담당자명");
// 발신자 담당자 메일주소
statement.setSenderEmail("test@test.com");
// 발신자 담당자 연락처
statement.setSenderTEL("070-7070-0707");
// 발신자 담당자 휴대폰번호
statement.setSenderHP("010-000-2222");
/*********************************************************************
* 수신자 정보
*********************************************************************/
// [필수] 수신자 사업자번호
statement.setReceiverCorpNum("8888888888");
// [필수] 수신자 상호
statement.setReceiverCorpName("수신자 상호");
// [필수] 수신자 대표자명
statement.setReceiverCEOName("수신자 대표자 성명");
// 수신자 주소
statement.setReceiverAddr("수신자 주소");
// 수신자 종목
statement.setReceiverBizClass("수신자 종목");
// 수신자 업태
statement.setReceiverBizType("수신자 업태");
// 수신자 담당자명
statement.setReceiverContactName("수신자 담당자명");
// 수신자 메일주소
// 팝빌 개발환경에서 테스트하는 경우에도 안내 메일이 전송되므로,
// 실제 거래처의 메일주소가 기재되지 않도록 주의
statement.setReceiverEmail("test@receiver.com");
/*********************************************************************
* 전자명세서 기재정보
*********************************************************************/
// 공급가액 합계
statement.setSupplyCostTotal("400000");
// 세액 합계
statement.setTaxTotal("40000");
// 합계금액. 공급가액 + 세액
statement.setTotalAmount("440000");
// 기재상 일련번호 항목
statement.setSerialNum("123");
// 기재상 비고 항목
statement.setRemark1("비고1");
statement.setRemark2("비고2");
statement.setRemark3("비고3");
// 사업자등록증 이미지 첨부여부
statement.setBusinessLicenseYN(false);
// 통장사본 이미지 첨부여부
statement.setBankBookYN(false);
/*********************************************************************
* 전자명세서 품목항목
*********************************************************************/
statement.setDetailList(new ArrayList<StatementDetail>());
StatementDetail detail = new StatementDetail(); // 상세항목(품목) 배열
detail.setSerialNum((short) 1); // 일련번호, 1부터 순차기재
detail.setItemName("품명"); // 품목명
detail.setPurchaseDT("20190827"); // 거래일자
detail.setQty("1"); // 수량
detail.setSupplyCost("200000"); // 공급가액
detail.setTax("20000"); // 세액
statement.getDetailList().add(detail);
detail = new StatementDetail(); // 상세항목(품목) 배열
detail.setSerialNum((short) 2); // 일련번호 1부터 순차기재
detail.setItemName("품명"); // 품목명
detail.setPurchaseDT("20190827"); // 거래일자
detail.setQty("1"); // 수량
detail.setSupplyCost("200000"); // 공급가액
detail.setTax("20000"); // 세액
statement.getDetailList().add(detail);
/*********************************************************************
* 전자명세서 추가속성
* - 추가속성에 관한 자세한 사항은 "[전자명세서 API 연동매뉴얼] >
* 5.2. 기본양식 추가속성 테이블"을 참조하시기 바랍니다.
*********************************************************************/
Map<String, String> propertyBag = new HashMap<String, String>();
propertyBag.put("Balance", "15000"); // 전잔액
propertyBag.put("Deposit", "5000"); // 입금액
propertyBag.put("CBalance", "20000"); // 현잔액
statement.setPropertyBag(propertyBag);
try {
Response response = statementService.registIssue(corpNum, statement, Memo, testUserID, emailSubject);
m.addAttribute("Response", response);
} catch (PopbillException e) {
// 예외 발생 시, e.getCode() 로 오류 코드를 확인하고, e.getMessage()로 오류 메시지를 확인합니다.
System.out.println("오류 코드" + e.getCode());
System.out.println("오류 메시지" + e.getMessage());
}
return "response";
}
}
② Add a page("src/main/webapp/WEB-INF/views/response.jsp") to output the result (code, message) of calling functions.
<%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c" %>
<%@ page pageEncoding="UTF-8" contentType="text/html; charset=UTF-8"%>
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8"/>
<title>Popbill SDK Response</title>
</head>
<body>
<p>Response.code : ${Response.code}</p>
<p>Response.message : ${Response.message}</p>
</body>
</html>
③ Call web-browser to check the result.
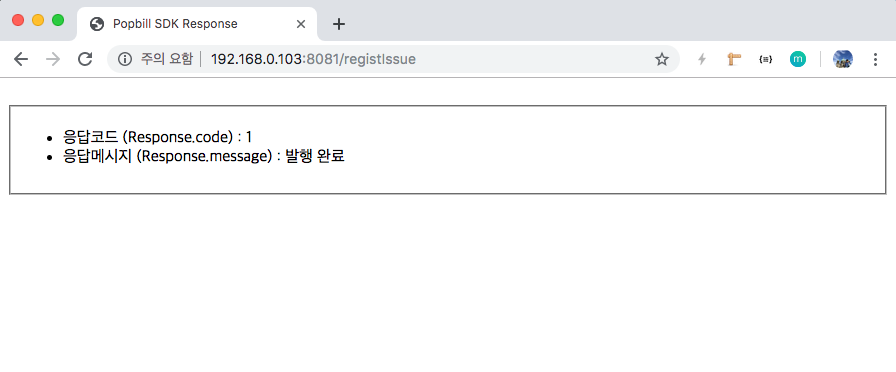
1. Add POPBiLL SDK CentOS 6.8Tomcat v7JDK v1.6
① Unzip the downloaded SDK example codes. [POPBiLL SDK Library - Java JSP Example]
② Copy 3 jar files below and Paste to library folder.

③ Create a file "$CATALINA_HOME/webapps/ROOT/common.jsp" to configure e-Tax invoice service backgrounds. Then, update the authentication information, 'LinkID' and 'SecretKey'.
Must change values of 'LinkID' and 'SecretKey' with the API Key issued by POPBiLL.
<jsp:useBean id="statementService" scope="application" class="com.popbill.api.statement.StatementServiceImp" />
<%-- 링크아이디 --%>
<jsp:setProperty name="statementService" property="linkID" value="TESTER" />
<%-- 비밀키, 사용자 인증에 사용되는 정보이므로 유출에 주의 --%>
<jsp:setProperty name="statementService" property="secretKey" value="SwWxqU+0TErBXy/9TVjIPEnI0VTUMMSQZtJf3Ed8q3I=" />
<%-- 연동환경 설정값, 개발용(true), 상업용(false) --%>
<jsp:setProperty name="statementService" property="test" value="true" />
<%-- 인증토큰 발급 IP 제한 On/Off, ture -제한기능 사용(기본값-권장), false-제한기능 미사용 --%>
<jsp:setProperty name="statementService" property="IPRestrictOnOff" value="true" />
<%-- 팝빌 API 서비스 고정 IP 사용여부, true-사용, false-미사용, 기본값(false) --%>
<jsp:setProperty name="statementService" property="useStaticIP" value="false"/>
2. Implement Function 'RegistIssue'
① Create file “$CATALINA_HOME/webapps/ROOT/registIssue.jsp” and Add codes like as below.
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8" />
<title>Popbill Statement Example</title>
</head>
<%@ include file="common.jsp" %>
<%@page import="java.util.Map"%>
<%@page import="java.util.HashMap"%>
<%@page import="java.util.ArrayList"%>
<%@page import="com.popbill.api.Response"%>
<%@page import="com.popbill.api.PopbillException"%>
<%@page import="com.popbill.api.statement.Statement"%>
<%@page import="com.popbill.api.statement.StatementDetail"%>
<%
/*
* 1건의 전자명세서를 즉시발행 처리합니다.
*/
// 팝빌회원 사업자번호
String testCorpNum = "1234567890";
// 팝빌회원 아이디
String testUserID = "testkorea";
// 메모
String memo = "즉시발행 메모 ";
// 발행안내 메일 제목, 미기재시 기본 양식으로 전송
String emailSubject = "메일제목 테스트";
/***************************************************************************
* 전자명세서 정보
****************************************************************************/
Statement statement = new Statement();
// [필수] 작성일자, 날짜형식(yyyyMMdd)
statement.setWriteDate("20191022");
// [필수] {영수, 청구} 중 기재
statement.setPurposeType("영수");
// [필수] 과세형태, {과세, 영세, 면세} 중 기재
statement.setTaxType("과세");
// 맞춤양식코드, 미기재시 기본양식으로 처리
statement.setFormCode("");
// [필수] 명세서 코드, [121 - 거래명세서], [122 - 청구서], [123 - 견적서], [124 - 발주서], [125 - 입금표], [126 - 영수증]
statement.setItemCode((short) 121);
// [필수] 문서번호, 최대 24자리 영문, 숫자, '-' 조합으로 구성
statement.setMgtKey("ABC0226-TEST001");
/***************************************************************************
* 발신자 정보
****************************************************************************/
// 발신자 사업자번호
statement.setSenderCorpNum(testCorpNum);
// 발신자 상호
statement.setSenderCorpName("발신자 상호");
// 발신자 주소
statement.setSenderAddr("발신자 주소");
// 발신자 대표자 성명
statement.setSenderCEOName("발신자 대표자 성명");
// 발신자 종사업장 식별번호
statement.setSenderTaxRegID("");
// 발신자 종목
statement.setSenderBizClass("종목");
// 발신자 업태
statement.setSenderBizType("업태");
// 발신자 담당자명
statement.setSenderContactName("발신자 담당자명");
// 발신자 담당자 메일주소
statement.setSenderEmail("test@test.com");
// 발신자 담당자 연락처
statement.setSenderTEL("070-7070-0707");
// 발신자 담당자 휴대폰번호
statement.setSenderHP("010-000-2222");
/***************************************************************************
* 수신자 정보
****************************************************************************/
// 수신자 사업자번호
statement.setReceiverCorpNum("8888888888");
// 수신자 상호
statement.setReceiverCorpName("수신자 상호");
// 수신자 대표자성명
statement.setReceiverCEOName("수신자 대표자 성명");
// 수신자 주소
statement.setReceiverAddr("수신자 주소");
// 수신자 종목
statement.setReceiverBizClass("수신자 업종");
// 수신자 업태
statement.setReceiverBizType("수신자 업태");
// 수신자 담당자명
statement.setReceiverContactName("수신자 담당자명");
// 수신자 담당자 메일주소
// 팝빌 개발환경에서 테스트하는 경우에도 안내 메일이 전송되므로,
// 실제 거래처의 메일주소가 기재되지 않도록 주의
statement.setReceiverEmail("test@test.com");
// 수신자 담당자 연락처
statement.setReceiverTEL("010-111-1111");
// 수신자 담당자 휴대폰번호
statement.setReceiverHP("010-1234-1234");
/***************************************************************************
* 전자명세서 기재정보
****************************************************************************/
// [필수] 공급가액 합계
statement.setSupplyCostTotal("400000");
// [필수] 세액 합계
statement.setTaxTotal("40000");
// [필수] 합계금액. 공급가액 + 세액
statement.setTotalAmount("440000");
// 기재상 일련번호 항목
statement.setSerialNum("123");
// 기재상 비고 항목
statement.setRemark1("비고1");
statement.setRemark2("비고2");
statement.setRemark3("비고3");
// 사업자등록증 이미지 첨부여부
statement.setBusinessLicenseYN(false);
// 통장사본 이미지 첨부여부
statement.setBankBookYN(false);
// 상세항목(품목) 배열
statement.setDetailList(new ArrayList<StatementDetail>());
StatementDetail detail = new StatementDetail();
detail.setSerialNum((short) 1); // 일련번호, 1부터 순차기재
detail.setItemName("품명"); // 품목명
detail.setPurchaseDT("20191022"); // 거래일자
detail.setQty("1"); // 수량
detail.setSupplyCost("200000"); // 공급가액
detail.setTax("20000"); // 세액
statement.getDetailList().add(detail);
detail = new StatementDetail(); // 상세항목(품목) 배열
detail.setSerialNum((short) 2); // 일련번호 1부터 순차기재
detail.setItemName("품명"); // 품목명
detail.setPurchaseDT("20191022"); // 거래일자
detail.setQty("1"); // 수량
detail.setSupplyCost("200000"); // 공급가액
detail.setTax("20000"); // 세액
statement.getDetailList().add(detail);
/***************************************************************************
* 추가속성 정보
* - 추가속성에 관한 자세한 사항은 전자명세서 API 연동매뉴얼 > 부록 > 기본양식 추가속성 테이블
* https://docs.popbill.com/en/statement/propertyBag?lang=java을 참조하시기 바랍니다.
****************************************************************************/
Map<String, String> propertyBag = new HashMap<String, String>();
propertyBag.put("Balance", "15000"); // 전잔액
propertyBag.put("Deposit", "5000"); // 입금액
propertyBag.put("CBalance", "20000"); // 현잔액
statement.setPropertyBag(propertyBag);
Response CheckResponse = null;
try {
CheckResponse = statementService.registIssue(testCorpNum, statement, memo, testUserID, emailSubject);
} catch (PopbillException pe) {
//적절한 오류 처리를 합니다. pe.getCode() 로 오류코드를 확인하고, pe.getMessage()로 관련 오류메시지를 확인합니다.
System.out.println("오류코드 " + pe.getCode());
System.out.println("오류메시지 " + pe.getMessage());
throw pe;
}
%>
<body>
<p>Response</p>
<br/>
<fieldset>
<legend>전자명세서 즉시발행</legend>
<ul>
<li>응답코드 (Response.code) : <%=CheckResponse.getCode()%></li>
<li>응답메시지 (Response.message) : <%=CheckResponse.getMessage()%></li>
</ul>
</fieldset>
</body>
</html>
② Call web-browser to check the result
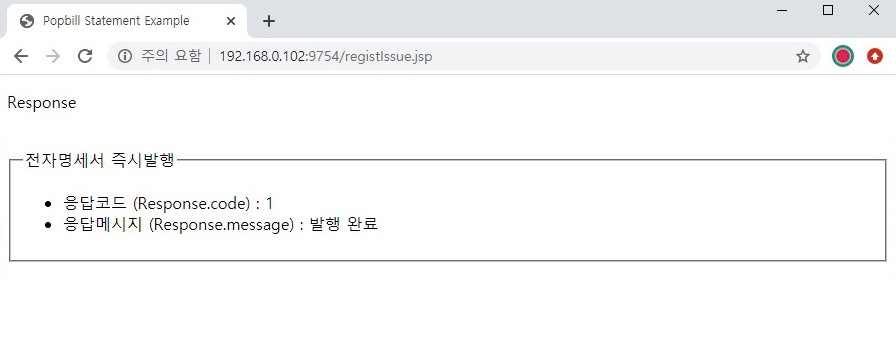
1. Add Popbill SpringBoot Starter using Gradle 
① Add dependency information to file "build.gradle" of SpringBoot project and Refresh..
※ POPBiLL SpringBoot Starter supports to SpringBoot version 1.0 and later and POPBiLL Java SDK AutoConfiguration.
dependencies {
implementation 'kr.co.linkhub:popbill-spring-boot-starter:1.4.0'
}
② Add the code below to file “appllication.yml” for setup SDK configuration.
Must change values of ‘LinkID’ and ‘SecretKey’ with the API Key issued by POPBiLL.
popbill:
#링크아이디
linkId: TESTER
#비밀키
secretKey: SwWxqU+0TErBXy/9TVjIPEnI0VTUMMSQZtJf3Ed8q3I=
#연동환경 설정값 true(개발용), false(상업용)
isTest: true
#인증토큰 아이피 제한 기능 사용여부 true(사용-권장), false(미사용)
isIpRestrictOnOff: true
#팝빌 API 서비스 고정 IP 사용여부 true(사용), false(미사용)
useStaticIp: false
#로컬시스템 시간 사용여부 true(사용-권장), false(미사용)
useLocalTimeYn: true
2. 전자명세서 즉시발행(RegistIssue) 기능 구현
① @Autowired 어노테이션을 이용하여 생성된 서비스 클래스 빈 객체를 주입 받은 후 registIssue 함수 코드를 추가합니다.
import java.util.ArrayList;
import java.util.HashMap;
import java.util.Map;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import com.popbill.api.PopbillException;
import com.popbill.api.SMTIssueResponse;
import com.popbill.api.StatementService;
import com.popbill.api.statement.Statement;
import com.popbill.api.statement.StatementDetail;
@Controller
@RequestMapping(value = "StatementService")
public class StatementServiceController {
@Autowired
private StatementService statementService;
@RequestMapping(value = "registIssue", method = RequestMethod.GET)
public String registIssue(Model m) {
// 발행자 사업자번호
String corpNum = "1234567890";
// 팝빌회원 아이디
String testUserID = "testkorea";
// 발행안내 메일 제목, 미기재시 기본 양식으로 전송
String emailSubject = "메일제목 테스트";
// 메모
String Memo = "전자명세서 즉시발행 메모";
// 전자명세서 정보 객체
Statement statement = new Statement();
// [필수] 작성일자, 형태 yyyyMMdd
statement.setWriteDate("20220101");
// [필수] {영수, 청구, 없음} 중 기재
statement.setPurposeType("영수");
// [필수] {과세, 영세, 면세} 중 기재
statement.setTaxType("과세");
// 맞춤양식코드, 미기재시 기본양식으로 처리
statement.setFormCode("");
// [필수] 명세서 코드, [121 - 거래명세서], [122 - 청구서], [123 - 견적서], [124 - 발주서], [125 - 입금표], [126 - 영수증]
statement.setItemCode((short) 121);
// [필수] 문서번호, 최대 24자리 영문, 숫자, '-', '_'로 구성
statement.setMgtKey("20220101-001");
/*********************************************************************
* 발신자 정보
*********************************************************************/
// [필수] 발신자 사업자번호
statement.setSenderCorpNum(corpNum);
// [필수] 발신자 상호
statement.setSenderCorpName("발신자 상호");
// 발신자 주소
statement.setSenderAddr("발신자 주소");
// [필수] 발신자 대표자 성명
statement.setSenderCEOName("발신자 대표자 성명");
// 발신자 종사업장 식별번호, 숫자 4자리, 필요시 기재
statement.setSenderTaxRegID("");
// 발신자 종목
statement.setSenderBizClass("업종");
// 발신자 업태
statement.setSenderBizType("업태");
// 발신자 담당자명
statement.setSenderContactName("발신자 담당자명");
// 발신자 담당자 메일주소
statement.setSenderEmail("test@test.com");
// 발신자 담당자 연락처
statement.setSenderTEL("070-7070-0707");
// 발신자 담당자 휴대폰번호
statement.setSenderHP("010-000-2222");
/*********************************************************************
* 수신자 정보
*********************************************************************/
// [필수] 수신자 사업자번호
statement.setReceiverCorpNum("8888888888");
// [필수] 수신자 상호
statement.setReceiverCorpName("수신자 상호");
// [필수] 수신자 대표자명
statement.setReceiverCEOName("수신자 대표자 성명");
// 수신자 주소
statement.setReceiverAddr("수신자 주소");
// 수신자 종목
statement.setReceiverBizClass("수신자 종목");
// 수신자 업태
statement.setReceiverBizType("수신자 업태");
// 수신자 담당자명
statement.setReceiverContactName("수신자 담당자명");
// 수신자 메일주소
// 팝빌 개발환경에서 테스트하는 경우에도 안내 메일이 전송되므로,
// 실제 거래처의 메일주소가 기재되지 않도록 주의
statement.setReceiverEmail("test@receiver.com");
/*********************************************************************
* 전자명세서 기재정보
*********************************************************************/
// 공급가액 합계
statement.setSupplyCostTotal("400000");
// 세액 합계
statement.setTaxTotal("40000");
// 합계금액. 공급가액 + 세액
statement.setTotalAmount("440000");
// 기재상 일련번호 항목
statement.setSerialNum("123");
// 기재상 비고 항목
statement.setRemark1("비고1");
statement.setRemark2("비고2");
statement.setRemark3("비고3");
// 사업자등록증 이미지 첨부여부
statement.setBusinessLicenseYN(false);
// 통장사본 이미지 첨부여부
statement.setBankBookYN(false);
/*********************************************************************
* 전자명세서 품목항목
*********************************************************************/
statement.setDetailList(new ArrayList<StatementDetail>());
StatementDetail detail = new StatementDetail(); // 상세항목(품목) 배열
detail.setSerialNum((short) 1); // 일련번호, 1부터 순차기재
detail.setItemName("품명"); // 품목명
detail.setPurchaseDT("20211125"); // 거래일자
detail.setQty("1"); // 수량
detail.setSupplyCost("200000"); // 공급가액
detail.setTax("20000"); // 세액
statement.getDetailList().add(detail);
detail = new StatementDetail(); // 상세항목(품목) 배열
detail.setSerialNum((short) 2); // 일련번호 1부터 순차기재
detail.setItemName("품명"); // 품목명
detail.setPurchaseDT("20211125"); // 거래일자
detail.setQty("1"); // 수량
detail.setSupplyCost("200000"); // 공급가액
detail.setTax("20000"); // 세액
statement.getDetailList().add(detail);
/*********************************************************************
* 전자명세서 추가속성
*********************************************************************/
Map<String, String> propertyBag = new HashMap<String, String>();
propertyBag.put("Balance", "15000"); // 전잔액
propertyBag.put("Deposit", "5000"); // 입금액
propertyBag.put("CBalance", "20000"); // 현잔액
statement.setPropertyBag(propertyBag);
try {
SMTIssueResponse response = statementService.registIssue(corpNum, statement, Memo, testUserID, emailSubject);
m.addAttribute("Response", response);
} catch (PopbillException e) {
// 예외 발생 시, e.getCode() 로 오류 코드를 확인하고, e.getMessage()로 오류 메시지를 확인합니다.
System.out.println("오류 코드" + e.getCode());
System.out.println("오류 메시지" + e.getMessage());
}
return "response";
}
}
② 함수 호출결과 코드와 메시지를 매핑하는 "src/main/resources/templates/response.html" 페이지를 추가합니다.
<html xmlns:th="http://www.thymeleaf.org"">
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8"/>
<title>Popbill SDK Response</title>
</head>
<body>
<ul>
<li>응답코드 (Response.code) : <span th:text="${Response.code}"></span></li>
<li>응답메시지 (Response.message) : <span th:text="${Response.message}"></span></li>
<li>팝빌 승인번호 (Response.invoiceNum) : <span th:text="${Response.invoiceNum}"></span></li>
</ul>
</body>
</html>
③ 웹브라우저 페이지를 호출하여 함수호출 결과를 확인합니다.
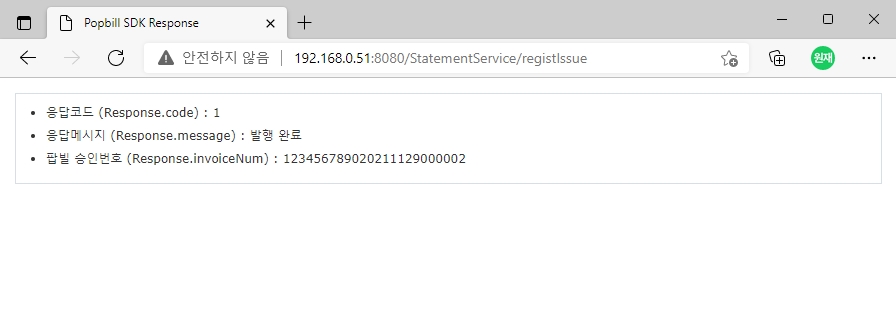
Business Contact
For more help with POPBiLL, try these resources :
T. +82 70-7998-7117E. global@linkhubcorp.com