Tutorial for POPBiLL e-Tax invoice
It is an example to implement SDK function 'RegistIssue'(To issue an e-Tax invoice) using POPBiLL Java SDK.
- SpringMVC
- SpringBoot
- JSP
1. Add POPBiLL SDK using Maven JDK v1.6Tomcat v7STS v3.6.4
① To add POPBiLL Java SDK, input the dependency information to file "pom.xml" and update Maven.
<dependency>
<groupId>kr.co.linkhub</groupId>
<artifactId>popbill-sdk</artifactId>
<version>1.57.1</version>
</dependency>
② Add a class of e-Tax invoice as bean of Spring. Update file "servlet-context.xml" referring codes below.
Must change values of LinkID and SecretKey with the API Key issued by POPBiLL.
<?xml version="1.0" encoding="UTF-8"?>
<beans:beans xmlns="http://www.springframework.org/schema/mvc"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:beans="http://www.springframework.org/schema/beans"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:util="http://www.springframework.org/schema/util"
xsi:schemaLocation="http://www.springframework.org/schema/mvc http://www.springframework.org/schema/mvc/spring-mvc.xsd
http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context.xsd
http://www.springframework.org/schema/util http://www.springframework.org/schema/util/spring-util.xsd">
<!-- DispatcherServlet Context: defines this servlet's request-processing infrastructure -->
<!-- Enables the Spring MVC @Controller programming model -->
<annotation-driven/>
<!-- Handles HTTP GET requests for /resources/** by efficiently serving up static resources in the ${webappRoot}/resources directory -->
<resources mapping="/resources/**" location="/resources/"/>
<!-- Resolves views selected for rendering by @Controllers to .jsp resources in the /WEB-INF/views directory -->
<beans:bean class="org.springframework.web.servlet.view.InternalResourceViewResolver">
<beans:property name="prefix" value="/WEB-INF/views/"/>
<beans:property name="suffix" value=".jsp"/>
</beans:bean>
<context:component-scan base-package="com.popbill.example"/>
<util:properties id="EXAMPLE_CONFIG">
<!-- 링크아이디 -->
<beans:prop key="LinkID">TESTER</beans:prop>
<!-- 비밀키 -->
<beans:prop key="SecretKey">SwWxqU+0TErBXy/9TVjIPEnI0VTUMMSQZtJf3Ed8q3I=</beans:prop>
<!-- 연동환경 설정값 true(개발용), false(상업용) -->
<beans:prop key="IsTest">true</beans:prop>
<!-- 인증토큰 아이피 제한 기능 사용여부 권장(true) -->
<beans:prop key="IsIPRestrictOnOff">true</beans:prop>
<!-- 팝빌 API 서비스 고정 IP 사용여부, true-사용, false-미사용, 기본값(false) -->
<beans:prop key="UseStaticIP">false</beans:prop>
</util:properties>
<beans:beans>
<!-- e-Tax invoice Service Implementation Bean registration. -->
<beans:bean id="taxinvoiceService" class="com.popbill.api.taxinvoice.TaxinvoiceServiceImp">
<beans:property name="linkID" value="#{EXAMPLE_CONFIG.LinkID}"/>
<beans:property name="secretKey" value="#{EXAMPLE_CONFIG.SecretKey}"/>
<beans:property name="test" value="#{EXAMPLE_CONFIG.IsTest}"/>
<beans:property name="IPRestrictOnOff" value="#{EXAMPLE_CONFIG.IsIPRestrictOnOff}"/>
<beans:property name="useStaticIP" value="#{EXAMPLE_CONFIG.UseStaticIP}"/>
</beans:bean>
</beans:beans>
</beans:beans>
2. Implement Function 'RegistIssue'
① Add @Autowired annotation and registIssue code to create the object of service class bean.
import java.util.ArrayList;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import com.popbill.api.IssueResponse;
import com.popbill.api.PopbillException;
import com.popbill.api.TaxinvoiceService;
import com.popbill.api.taxinvoice.Taxinvoice;
import com.popbill.api.taxinvoice.TaxinvoiceAddContact;
import com.popbill.api.taxinvoice.TaxinvoiceDetail;
@Controller
public class TaxinvoiceServiceExample {
@Autowired
private TaxinvoiceService taxinvoiceService;
@RequestMapping(value = "registIssue", method = RequestMethod.GET)
public String registIssue(Model m) {
// 세금계산서 정보 객체
Taxinvoice taxinvoice = new Taxinvoice();
// 작성일자, 날짜형식(yyyyMMdd)
taxinvoice.setWriteDate("20190827");
// 과금방향, [정과금, 역과금] 중 선택기재, "역과금"은 역발행세금계산서 발행에만 가능
taxinvoice.setChargeDirection("정과금");
// 발행유형, [정발행, 역발행, 위수탁] 중 기재
taxinvoice.setIssueType("정발행");
// [영수, 청구, 없음] 중 기재
taxinvoice.setPurposeType("영수");
// 발행시점, [직접발행, 승인시자동발행] 중 기재
taxinvoice.setIssueTiming("직접발행");
// 과세형태, [과세, 영세, 면세] 중 기재
taxinvoice.setTaxType("과세");
/*********************************************************************
* 공급자 정보
*********************************************************************/
// 공급자 사업자번호
taxinvoice.setInvoicerCorpNum("1234567890");
// 공급자 종사업장 식별번호, 필요시 기재. 형식은 숫자 4자리.
taxinvoice.setInvoicerTaxRegID("");
// 공급자 상호
taxinvoice.setInvoicerCorpName("공급자 상호");
// 공급자 문서번호, 1~24자리 (숫자, 영문, '-', '_') 조합으로 사업자 별로 중복되지 않도록 구성
taxinvoice.setInvoicerMgtKey("20190827-001");
// 공급자 대표자성명
taxinvoice.setInvoicerCEOName("공급자 대표자 성명");
// 공급자 주소
taxinvoice.setInvoicerAddr("공급자 주소");
// 공급자 종목
taxinvoice.setInvoicerBizClass("공급자 업종");
// 공급자 업태
taxinvoice.setInvoicerBizType("공급자 업태,업태2");
// 공급자 담당자 성명
taxinvoice.setInvoicerContactName("공급자 담당자명");
// 공급자 담당자 메일주소
taxinvoice.setInvoicerEmail("test@test.com");
// 공급자 담당자 연락처
taxinvoice.setInvoicerTEL("070-7070-0707");
// 공급자 담당자 휴대폰번호
taxinvoice.setInvoicerHP("010-000-2222");
// 발행 안내문자메시지 전송여부
// - 전송시 포인트 차감되며, 전송실패시 환불처리
taxinvoice.setInvoicerSMSSendYN(false);
/*********************************************************************
* 공급받는자 정보
*********************************************************************/
// 공급받는자 구분, [사업자, 개인, 외국인] 중 기재
taxinvoice.setInvoiceeType("사업자");
// 공급받는자 사업자번호, '-' 제외 10자리
taxinvoice.setInvoiceeCorpNum("8888888888");
// 공급받는자 상호
taxinvoice.setInvoiceeCorpName("공급받는자 상호");
// [역발행시 필수] 공급받는자 문서번호, 1~24자리까지 사업자번호별 중복없는 고유번호 할당
taxinvoice.setInvoiceeMgtKey("");
// 공급받는자 대표자 성명
taxinvoice.setInvoiceeCEOName("공급받는자 대표자 성명");
// 공급받는자 주소
taxinvoice.setInvoiceeAddr("공급받는자 주소");
// 공급받는자 종목
taxinvoice.setInvoiceeBizClass("공급받는자 업종");
// 공급받는자 업태
taxinvoice.setInvoiceeBizType("공급받는자 업태");
// 공급받는자 담당자명
taxinvoice.setInvoiceeContactName1("공급받는자 담당자명");
// 공급받는자 담당자 메일주소
// 팝빌 개발환경에서 테스트하는 경우에도 안내 메일이 전송되므로,
// 실제 거래처의 메일주소가 기재되지 않도록 주의
taxinvoice.setInvoiceeEmail1("test@invoicee.com");
// 공급받는자 담당자 연락처
taxinvoice.setInvoiceeTEL1("070-111-222");
// 공급받는자 담당자 휴대폰번호
taxinvoice.setInvoiceeHP1("010-111-222");
// 역발행시 안내문자메시지 전송여부
// - 전송시 포인트 차감되며, 전송실패시 환불처리
taxinvoice.setInvoiceeSMSSendYN(false);
/*********************************************************************
* 세금계산서 기재정보
*********************************************************************/
// [필수] 공급가액 합계
taxinvoice.setSupplyCostTotal("100000");
// [필수] 세액 합계
taxinvoice.setTaxTotal("10000");
// [필수] 합계금액, 공급가액 + 세액
taxinvoice.setTotalAmount("110000");
// 기재 상 일련번호
taxinvoice.setSerialNum("123");
// 기재 상 현금
taxinvoice.setCash("");
// 기재 상 수표
taxinvoice.setChkBill("");
// 기재 상 어음
taxinvoice.setNote("");
// 기재 상 외상미수금
taxinvoice.setCredit("");
// 기재 상 비고
taxinvoice.setRemark1("비고1");
taxinvoice.setRemark2("비고2");
taxinvoice.setRemark3("비고3");
taxinvoice.setKwon((short) 1);
taxinvoice.setHo((short) 1);
// 사업자등록증 이미지 첨부여부
taxinvoice.setBusinessLicenseYN(false);
// 통장사본 이미지 첨부여부
taxinvoice.setBankBookYN(false);
/*********************************************************************
* 수정세금계산서 정보 (수정세금계산서 작성시 기재)
* - 수정세금계산서 관련 정보는 연동매뉴얼 또는 개발가이드 링크 참조
& - [참고] 수정세금계산서 작성방법 안내 [http://blog.linkhubcorp.com/650]
*********************************************************************/
// [수정세금계산서 작성시 필수] 수정사유코드, 수정사유에 따라 1~6 중 선택기재.
taxinvoice.setModifyCode(null);
// [수정세금계산서 작성시 필수] 원본세금계산서의 국세청승인번호 기재
taxinvoice.setOrgNTSConfirmNum("");
/*********************************************************************
* 상세항목(품목) 정보
*********************************************************************/
taxinvoice.setDetailList(new ArrayList<TaxinvoiceDetail>());
// 상세항목 객체
TaxinvoiceDetail detail = new TaxinvoiceDetail();
detail.setSerialNum((short) 1); // 일련번호, 1부터 순차기재
detail.setPurchaseDT("20190827"); // 거래일자
detail.setItemName("품목명");
detail.setSpec("규격");
detail.setQty("1"); // 수량
detail.setUnitCost("50000"); // 단가
detail.setSupplyCost("50000"); // 공급가액
detail.setTax("5000"); // 세액
detail.setRemark("품목비고");
taxinvoice.getDetailList().add(detail);
detail = new TaxinvoiceDetail();
detail.setSerialNum((short) 2); // 일련번호, 1부터 순차기재
detail.setPurchaseDT("20190827"); // 거래일자
detail.setItemName("품목명2");
detail.setSpec("규격");
detail.setQty("1"); // 수량
detail.setUnitCost("50000"); // 단가
detail.setSupplyCost("50000"); // 공급가액
detail.setTax("5000"); // 세액
detail.setRemark("품목비고2");
taxinvoice.getDetailList().add(detail);
/*********************************************************************
* 추가담당자 정보
*********************************************************************/
taxinvoice.setAddContactList(new ArrayList<TaxinvoiceAddContact>());
TaxinvoiceAddContact addContact = new TaxinvoiceAddContact();
addContact.setSerialNum(1);
addContact.setContactName("추가 담당자명");
addContact.setEmail("test2@test.com");
taxinvoice.getAddContactList().add(addContact);
// 거래명세서 동시작성여부
Boolean WriteSpecification = false;
// 거래명세서 문서번호
String DealInvoiceKey = null;
// 즉시발행 메모
String Memo = "즉시발행 메모";
// 지연발행 강제여부
// 발행마감일이 지난 세금계산서를 발행하는 경우, 가산세가 부과될 수 있습니다.
// 가산세가 부과되더라도 발행을 해야하는 경우에는 forceIssue의 값을
// true로 선언하여 발행(Issue API)를 호출하시면 됩니다.
Boolean ForceIssue = false;
try {
IssueResponse response = taxinvoiceService.registIssue("1234567890",
taxinvoice, WriteSpecification, Memo, ForceIssue, DealInvoiceKey);
m.addAttribute("Response", response);
} catch (PopbillException e) {
// 예외 발생 시, e.getCode() 로 오류 코드를 확인하고, e.getMessage()로 오류 메시지를 확인합니다.
System.out.println("오류 코드" + e.getCode());
System.out.println("오류 메시지" + e.getMessage());
}
return "response";
}
}
② Add a page("src/main/webapp/WEB-INF/views/response.jsp") to output the result (code, message) of calling functions.
<%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c" %>
<%@ page pageEncoding="UTF-8" contentType="text/html; charset=UTF-8"%>
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8"/>
<title>Popbill SDK Response</title>
</head>
<body>
<p>응답코드 (Response.code) : ${Response.code}</p>
<p>응답메시지 (Response.message) : ${Response.message}</p>
<p>국세청승인번호 (Response.ntsConfirmNum) : ${Response.ntsConfirmNum}</p>
</body>
</html>
③ Call web-browser to check the result.
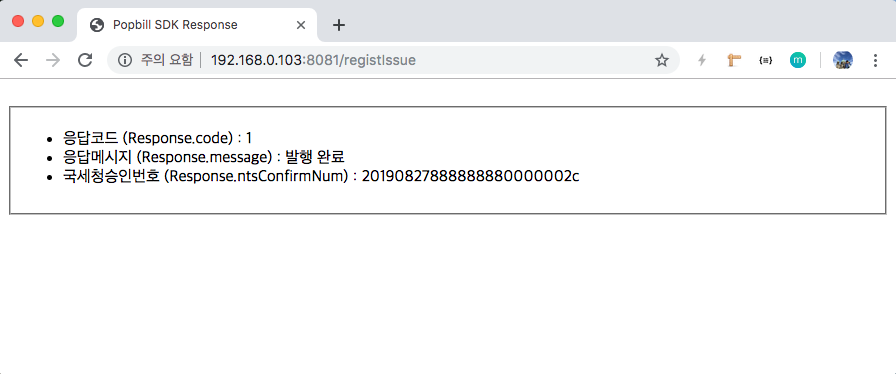
1. Add POPBiLL SDK CentOS 6.8Tomcat v7JDK v1.6
① Unzip the downloaded SDK example codes. [POPBiLL SDK Library - Java JSP Example]
② Copy 3 jar files below and Paste to library folder.

③ Create a file "$CATALINA_HOME/webapps/ROOT/common.jsp" to configure e-Tax invoice service backgrounds. Then, update the authentication information, 'LinkID' and 'SecretKey'.
Must change values of 'LinkID' and 'SecretKey' with the API Key issued by POPBiLL.
<%-- 전자세금계산서 클래스 빈 생성 --%>
<jsp:useBean id="taxinvoiceService" scope="application" class="com.popbill.api.taxinvoice.TaxinvoiceServiceImp"/>
<%-- 링크아이디(LinkID), 연동신청시 발급받은 정보로 수정 --%>
<jsp:setProperty name="taxinvoiceService" property="linkID" value="TESTER"/>
<%-- 비밀키(SecretKey), 연동신청시 발급받은 정보로 수정 --%>
<jsp:setProperty name="taxinvoiceService" property="secretKey" value="SwWxqU+0TExEXy/9TVjKPExI2VTUMMSLZtJf3Ed8q3I="/>
<%-- 연동환경 설정값, true-개발용(테스트베드), false-상업용(실서비스) --%>
<jsp:setProperty name="taxinvoiceService" property="test" value="true"/>
<%-- 인증토큰 발급 IP 제한 On/Off, ture-제한기능 사용(기본값-권장), false-제한기능 미사용 --%>
<jsp:setProperty name="taxinvoiceService" property="IPRestrictOnOff" value="true"/>
<%-- 팝빌 API 서비스 고정 IP 사용여부, true-사용, false-미사용, 기본값(false) --%>
<jsp:setProperty name="taxinvoiceService" property="useStaticIP" value="false"/>
2. Implement Function 'RegistIssue'
① Create file "$CATALINA_HOME/webapps/ROOT/registIssue.jsp" and Add codes like as below.
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8" />
<title>Popbill Taxinvoice Example</title>
</head>
<%@ include file="common.jsp" %>
<%@page import="java.util.ArrayList"%>
<%@page import="com.popbill.api.IssueResponse"%>
<%@page import="com.popbill.api.PopbillException"%>
<%@page import="com.popbill.api.taxinvoice.Taxinvoice"%>
<%@page import="com.popbill.api.taxinvoice.TaxinvoiceDetail"%>
<%@page import="com.popbill.api.taxinvoice.TaxinvoiceAddContact"%>
<%
/*
* 1건의 세금계산서를 즉시발행 처리합니다.
* - 세금계산서 항목별 정보는 "[전자세금계산서 API 연동매뉴얼] > 4.1. (세금)계산서구성"을 참조하시기 바랍니다.
*/
// 팝빌회원 사업자번호
String testCorpNum = "1234567890";
// 거래명세서 동시작성 여부
Boolean writeSpecification = false;
// 메모
String memo = "즉시발행 메모";
// 지연발행 강제여부
// 발행마감일이 지난 세금계산서를 발행하는 경우, 가산세가 부과될 수 있습니다.
// 가산세가 부과되더라도 발행을 해야하는 경우에는 forceIssue의 값을
// true로 선언하여 발행(Issue API)를 호출하시면 됩니다.
Boolean forceIssue = false;
// 거래명세서 동시작성시 명세서 문서번호. 미기재시 공급자 문서번호로 동일하게 구성
String dealInvoiceKey = "";
/***************************************************************************
* 세금계산서 정보
****************************************************************************/
Taxinvoice taxinvoice = new Taxinvoice();
// 필수, 기재상 작성일자, 날짜형식(yyyyMMdd)
taxinvoice.setWriteDate("20200110");
// 발행유형, {정발행, 역발행, 위수탁} 중 기재
taxinvoice.setIssueType("정발행");
// 과금방향, {정과금, 역과금}, '역과금'은 역발행 세금계산서 발행시에만 이용가능
taxinvoice.setChargeDirection("정과금");
// 필수, {영수, 청구, 없음} 중 기재
taxinvoice.setPurposeType("영수");
// 필수, 과세형태, {과세, 영세, 면세} 중 기재
taxinvoice.setTaxType("과세");
/***************************************************************************
* 공급자 정보
****************************************************************************/
// 공급자 사업자번호, "-"제외
taxinvoice.setInvoicerCorpNum(testCorpNum);
// 종사업자 식별번호. 필요시 기재. 형식은 숫자 4자리.
taxinvoice.setInvoicerTaxRegID("");
// 공급자 상호
taxinvoice.setInvoicerCorpName("공급자 상호");
// 공급자 문서번호, 1~24자리 (숫자, 영문, '-', '_') 조합으로 사업자 별로 중복되지 않도록 구성
taxinvoice.setInvoicerMgtKey("20200110-TEST001");
// 공급자 대표자성명
taxinvoice.setInvoicerCEOName("공급자 대표자 성명");
// 공급자 주소
taxinvoice.setInvoicerAddr("공급자 주소");
// 공급자 종목
taxinvoice.setInvoicerBizClass("공급자 업종");
// 공급자 업태
taxinvoice.setInvoicerBizType("공급자 업태,업태2");
// 공급자 담당자명
taxinvoice.setInvoicerContactName("공급자 담당자명");
// 공급자 담당자 메일주소
taxinvoice.setInvoicerEmail("test@test.com");
// 공급자 담당자 연락처
taxinvoice.setInvoicerTEL("070-7070-0707");
// 공급자 휴대폰번호
taxinvoice.setInvoicerHP("010-000-2222");
// 발행시 안내문자 전송여부
taxinvoice.setInvoicerSMSSendYN(false);
/***************************************************************************
* 공급받는 정보
****************************************************************************/
// 공급받는자 구분 {사업자 , 개인 , 외국인} 중 기재
taxinvoice.setInvoiceeType("사업자");
// 공급받는자 사업자번호, '-' 제외 10자리
taxinvoice.setInvoiceeCorpNum("8888888888");
// 공급받는자 종사업장 식별번호, 필요시 숫자4자리 기재
taxinvoice.setInvoiceeTaxRegID("");
// 공급받는자 상호
taxinvoice.setInvoiceeCorpName("공급받는자 상호");
// 공급받는자 문서번호, 역발행시 필수
taxinvoice.setInvoiceeMgtKey("");
// 공급받는자 대표자성명
taxinvoice.setInvoiceeCEOName("공급받는자 대표자 성명");
// 공급받는자 주소
taxinvoice.setInvoiceeAddr("공급받는자 주소");
// 공급받는자 종목
taxinvoice.setInvoiceeBizClass("공급받는자 업종");
// 공급받는자 업태
taxinvoice.setInvoiceeBizType("공급받는자 업태");
// 공급받는자 담당자명
taxinvoice.setInvoiceeContactName1("공급받는자 담당자명");
// 공급받는자 메일주소
// 팝빌 개발환경에서 테스트하는 경우에도 안내 메일이 전송되므로,
// 실제 거래처의 메일주소가 기재되지 않도록 주의
taxinvoice.setInvoiceeEmail1("test@test.com");
// 공급받는자 연락처
taxinvoice.setInvoiceeTEL1("070-1234-1234");
// 공급받는자 휴대폰번호
taxinvoice.setInvoiceeHP1("010-000-1111");
/***************************************************************************
* 세금계산서 기재정보
****************************************************************************/
// 공급가액 합계
taxinvoice.setSupplyCostTotal("200000");
// 세액 합계
taxinvoice.setTaxTotal("20000");
// 합계금액. 공급가액 + 세액
taxinvoice.setTotalAmount("220000");
// 일련번호 항목
taxinvoice.setSerialNum("123");
// 현금
taxinvoice.setCash("");
// 수표
taxinvoice.setChkBill("");
// 어음
taxinvoice.setNote("");
// 외상미수금
taxinvoice.setCredit("");
taxinvoice.setRemark1("비고1");
taxinvoice.setRemark2("비고2");
taxinvoice.setRemark3("비고3");
// 기재상 '권' 항목, 최대값 32767
taxinvoice.setKwon((short) 1);
// 기재상 '호' 항목, 최대값 32767
taxinvoice.setHo((short) 1);
// 사업자등록증 이미지 첨부여부
taxinvoice.setBusinessLicenseYN(false);
// 통장사본 이미지 첨부여부
taxinvoice.setBankBookYN(false);
/***************************************************************************
* - 수정세금계산서 정보 (수정세금계산서 작성시에만 입력
* - 수정세금계산서 관련 정보는 연동매뉴얼 또는 개발가이드 링크 참조
****************************************************************************/
// 수정세금계산서 작성시 1~6까지 선택기재.
taxinvoice.setModifyCode(null);
// 수정세금계산서 작성시 원본세금계산서의 국세청승인번호 기재
taxinvoice.setOrgNTSConfirmNum("");
/***************************************************************************
* 상세항목(품목) 정보
****************************************************************************/
taxinvoice.setDetailList(new ArrayList<TaxinvoiceDetail>());
TaxinvoiceDetail detail = new TaxinvoiceDetail();
detail.setSerialNum((short) 1); // 일련번호
detail.setPurchaseDT("20190107"); // 거래일자
detail.setItemName("품목명1"); // 품목명
detail.setSpec("규격"); // 규격
detail.setQty("1"); // 수량
detail.setUnitCost("100000"); // 단가
detail.setSupplyCost("100000"); // 공급가액
detail.setTax("10000"); // 세액
detail.setRemark("품목비고"); // 비고
taxinvoice.getDetailList().add(detail);
detail = new TaxinvoiceDetail();
detail.setSerialNum((short) 2);
detail.setPurchaseDT("20190107");
detail.setItemName("품목명2");
detail.setSpec("규격");
detail.setQty("1");
detail.setUnitCost("100000");
detail.setSupplyCost("100000");
detail.setTax("10000");
detail.setRemark("품목비고");
taxinvoice.getDetailList().add(detail);
/***************************************************************************
* 추가담당자 정보
* - 세금계산서 발행 안내 메일을 다수의 담당자에게 전송할 경우 추가 담당자 정보를 추가하면
* 다수의 담당자에게 이메일을 전송할 수 있습니다.
****************************************************************************/
taxinvoice.setAddContactList(new ArrayList<TaxinvoiceAddContact>());
TaxinvoiceAddContact addContact = new TaxinvoiceAddContact();
addContact.setSerialNum(1);
addContact.setContactName("추가 담당자명");
addContact.setEmail("test2@test.com");
taxinvoice.getAddContactList().add(addContact);
addContact = new TaxinvoiceAddContact();
addContact.setSerialNum(2);
addContact.setContactName("추가 담당자명");
addContact.setEmail("test2@test.com");
taxinvoice.getAddContactList().add(addContact);
IssueResponse CheckResponse = null;
try {
CheckResponse = taxinvoiceService.registIssue(testCorpNum, taxinvoice, writeSpecification,
memo, forceIssue, dealInvoiceKey);
} catch (PopbillException pe) {
//적절한 오류 처리를 합니다. pe.getCode() 로 오류코드를 확인하고, pe.getMessage()로 관련 오류메시지를 확인합니다.
System.out.println("오류코드 " + pe.getCode());
System.out.println("오류메세지 " + pe.getMessage());
throw pe;
}
%>
<body>
<p>Response</p>
<br/>
<fieldset>
<legend>세금계산서 즉시발행</legend>
<ul>
<li>응답코드 (IssueResponse.code) : <%=CheckResponse.getCode()%></li>
<li>응답메시지 (IssueResponse.message) : <%=CheckResponse.getMessage()%></li>
<li>국세청 승인번호 (IssueResponse.ntsConfirmNum) : <%=CheckResponse.getNtsConfirmNum()%></li>
</ul>
</fieldset>
</body>
</html>
② Call web-browser to check the result
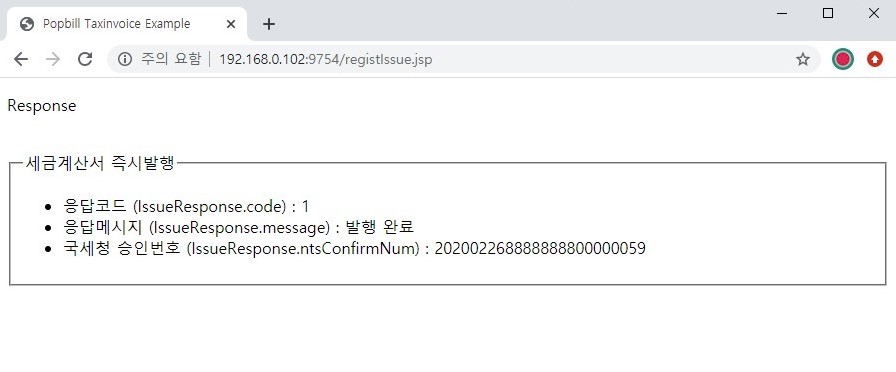
1. Add Popbill SpringBoot Starter using Gradle 
① Add dependency information to file "build.gradle" of SpringBoot project and Refresh..
※ POPBiLL SpringBoot Starter supports to SpringBoot version 1.0 and later and POPBiLL Java SDK AutoConfiguration.
dependencies {
implementation 'kr.co.linkhub:popbill-spring-boot-starter:1.4.0'
}
② Add the code below to file “appllication.yml” for setup SDK configuration.
Must change values of ‘LinkID’ and ‘SecretKey’ with the API Key issued by POPBiLL.
popbill:
#링크아이디
linkId: TESTER
#비밀키
secretKey: SwWxqU+0TErBXy/9TVjIPEnI0VTUMMSQZtJf3Ed8q3I=
#연동환경 설정값 true(개발용), false(상업용)
isTest: true
#인증토큰 아이피 제한 기능 사용여부 true(사용-권장), false(미사용)
isIpRestrictOnOff: true
#팝빌 API 서비스 고정 IP 사용여부 true(사용), false(미사용)
useStaticIp: false
#로컬시스템 시간 사용여부 true(사용-권장), false(미사용)
useLocalTimeYn: true
2. Implement Function 'RegistIssue'
① Add @Autowired annotation and registIssue code to create the object of service class bean.
import java.util.ArrayList;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import com.popbill.api.IssueResponse;
import com.popbill.api.PopbillException;
import com.popbill.api.TaxinvoiceService;
import com.popbill.api.taxinvoice.Taxinvoice;
import com.popbill.api.taxinvoice.TaxinvoiceAddContact;
import com.popbill.api.taxinvoice.TaxinvoiceDetail;
@Controller
public class TaxinvoiceServiceController {
@Autowired
private TaxinvoiceService taxinvoiceService;
@RequestMapping(value = "registIssue", method = RequestMethod.GET)
public String registIssue(Model m) {
// 세금계산서 정보 객체
Taxinvoice taxinvoice = new Taxinvoice();
// 작성일자, 날짜형식(yyyyMMdd)
taxinvoice.setWriteDate("20211123");
// 과금방향, [정과금, 역과금] 중 선택기재, "역과금"은 역발행세금계산서 발행에만 가능
taxinvoice.setChargeDirection("정과금");
// 발행유형, [정발행, 역발행, 위수탁] 중 기재
taxinvoice.setIssueType("정발행");
// [영수, 청구, 없음] 중 기재
taxinvoice.setPurposeType("영수");
// 발행시점, [직접발행, 승인시자동발행] 중 기재
taxinvoice.setIssueTiming("직접발행");
// 과세형태, [과세, 영세, 면세] 중 기재
taxinvoice.setTaxType("과세");
/*********************************************************************
* 공급자 정보
*********************************************************************/
// 공급자 사업자번호
taxinvoice.setInvoicerCorpNum("1234567890");
// 공급자 종사업장 식별번호, 필요시 기재. 형식은 숫자 4자리.
taxinvoice.setInvoicerTaxRegID("");
// 공급자 상호
taxinvoice.setInvoicerCorpName("공급자 상호");
// 공급자 문서번호, 1~24자리 (숫자, 영문, '-', '_') 조합으로 사업자 별로 중복되지 않도록 구성
taxinvoice.setInvoicerMgtKey("20211123-001");
// 공급자 대표자성명
taxinvoice.setInvoicerCEOName("공급자 대표자 성명");
// 공급자 주소
taxinvoice.setInvoicerAddr("공급자 주소");
// 공급자 종목
taxinvoice.setInvoicerBizClass("공급자 업종");
// 공급자 업태
taxinvoice.setInvoicerBizType("공급자 업태,업태2");
// 공급자 담당자 성명
taxinvoice.setInvoicerContactName("공급자 담당자명");
// 공급자 담당자 메일주소
taxinvoice.setInvoicerEmail("test@test.com");
// 공급자 담당자 연락처
taxinvoice.setInvoicerTEL("070-7070-0707");
// 공급자 담당자 휴대폰번호
taxinvoice.setInvoicerHP("010-000-2222");
// 발행 안내문자메시지 전송여부
// - 전송시 포인트 차감되며, 전송실패시 환불처리
taxinvoice.setInvoicerSMSSendYN(false);
/*********************************************************************
* 공급받는자 정보
*********************************************************************/
// 공급받는자 구분, [사업자, 개인, 외국인] 중 기재
taxinvoice.setInvoiceeType("사업자");
// 공급받는자 사업자번호, '-' 제외 10자리
taxinvoice.setInvoiceeCorpNum("8888888888");
// 공급받는자 상호
taxinvoice.setInvoiceeCorpName("공급받는자 상호");
// [역발행시 필수] 공급받는자 문서번호, 1~24자리까지 사업자번호별 중복없는 고유번호 할당
taxinvoice.setInvoiceeMgtKey("");
// 공급받는자 대표자 성명
taxinvoice.setInvoiceeCEOName("공급받는자 대표자 성명");
// 공급받는자 주소
taxinvoice.setInvoiceeAddr("공급받는자 주소");
// 공급받는자 종목
taxinvoice.setInvoiceeBizClass("공급받는자 업종");
// 공급받는자 업태
taxinvoice.setInvoiceeBizType("공급받는자 업태");
// 공급받는자 담당자명
taxinvoice.setInvoiceeContactName1("공급받는자 담당자명");
// 공급받는자 담당자 메일주소
// 팝빌 개발환경에서 테스트하는 경우에도 안내 메일이 전송되므로,
// 실제 거래처의 메일주소가 기재되지 않도록 주의
taxinvoice.setInvoiceeEmail1("test@invoicee.com");
// 공급받는자 담당자 연락처
taxinvoice.setInvoiceeTEL1("070-111-222");
// 공급받는자 담당자 휴대폰번호
taxinvoice.setInvoiceeHP1("010-111-222");
// 역발행시 안내문자메시지 전송여부
// - 전송시 포인트 차감되며, 전송실패시 환불처리
taxinvoice.setInvoiceeSMSSendYN(false);
/*********************************************************************
* 세금계산서 기재정보
*********************************************************************/
// [필수] 공급가액 합계
taxinvoice.setSupplyCostTotal("100000");
// [필수] 세액 합계
taxinvoice.setTaxTotal("10000");
// [필수] 합계금액, 공급가액 + 세액
taxinvoice.setTotalAmount("110000");
// 기재 상 일련번호
taxinvoice.setSerialNum("123");
// 기재 상 현금
taxinvoice.setCash("");
// 기재 상 수표
taxinvoice.setChkBill("");
// 기재 상 어음
taxinvoice.setNote("");
// 기재 상 외상미수금
taxinvoice.setCredit("");
// 기재 상 비고
taxinvoice.setRemark1("비고1");
taxinvoice.setRemark2("비고2");
taxinvoice.setRemark3("비고3");
taxinvoice.setKwon((short) 1);
taxinvoice.setHo((short) 1);
// 사업자등록증 이미지 첨부여부
taxinvoice.setBusinessLicenseYN(false);
// 통장사본 이미지 첨부여부
taxinvoice.setBankBookYN(false);
/*********************************************************************
* 수정세금계산서 정보 (수정세금계산서 작성시 기재)
* - 수정세금계산서 관련 정보는 연동매뉴얼 또는 개발가이드 링크 참조
& - [참고] 수정세금계산서 작성방법 안내 [http://blog.linkhubcorp.com/650]
*********************************************************************/
// [수정세금계산서 작성시 필수] 수정사유코드, 수정사유에 따라 1~6 중 선택기재.
taxinvoice.setModifyCode(null);
// [수정세금계산서 작성시 필수] 원본세금계산서의 국세청승인번호 기재
taxinvoice.setOrgNTSConfirmNum("");
/*********************************************************************
* 상세항목(품목) 정보
*********************************************************************/
taxinvoice.setDetailList(new ArrayList<TaxinvoiceDetail>());
// 상세항목 객체
TaxinvoiceDetail detail = new TaxinvoiceDetail();
detail.setSerialNum((short) 1); // 일련번호, 1부터 순차기재
detail.setPurchaseDT("20211123"); // 거래일자
detail.setItemName("품목명");
detail.setSpec("규격");
detail.setQty("1"); // 수량
detail.setUnitCost("50000"); // 단가
detail.setSupplyCost("50000"); // 공급가액
detail.setTax("5000"); // 세액
detail.setRemark("품목비고");
taxinvoice.getDetailList().add(detail);
detail = new TaxinvoiceDetail();
detail.setSerialNum((short) 2); // 일련번호, 1부터 순차기재
detail.setPurchaseDT("20211123"); // 거래일자
detail.setItemName("품목명2");
detail.setSpec("규격");
detail.setQty("1"); // 수량
detail.setUnitCost("50000"); // 단가
detail.setSupplyCost("50000"); // 공급가액
detail.setTax("5000"); // 세액
detail.setRemark("품목비고2");
taxinvoice.getDetailList().add(detail);
/*********************************************************************
* 추가담당자 정보
*********************************************************************/
taxinvoice.setAddContactList(new ArrayList<TaxinvoiceAddContact>());
TaxinvoiceAddContact addContact = new TaxinvoiceAddContact();
addContact.setSerialNum(1);
addContact.setContactName("추가 담당자명");
addContact.setEmail("test2@test.com");
taxinvoice.getAddContactList().add(addContact);
// 거래명세서 동시작성여부
Boolean WriteSpecification = false;
// 거래명세서 문서번호
String DealInvoiceKey = null;
// 즉시발행 메모
String Memo = "즉시발행 메모";
// 지연발행 강제여부
// 발행마감일이 지난 세금계산서를 발행하는 경우, 가산세가 부과될 수 있습니다.
// 가산세가 부과되더라도 발행을 해야하는 경우에는 forceIssue의 값을
// true로 선언하여 발행(Issue API)를 호출하시면 됩니다.
Boolean ForceIssue = false;
try {
IssueResponse response = taxinvoiceService.registIssue("1234567890",
taxinvoice, WriteSpecification, Memo, ForceIssue, DealInvoiceKey);
m.addAttribute("Response", response);
} catch (PopbillException e) {
// 예외 발생 시, e.getCode() 로 오류 코드를 확인하고, e.getMessage()로 오류 메시지를 확인합니다.
System.out.println("오류 코드" + e.getCode());
System.out.println("오류 메시지" + e.getMessage());
}
return "response";
}
}
② Add a page(“src/main/resources/templates/response.html") to output the result(code, message) of calling functions.
<html xmlns:th="http://www.thymeleaf.org"">
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8"/>
<title>Popbill SDK Response</title>
</head>
<body>
<fieldset>
<ul>
<li>응답코드 (Response.code) : <span th:text="${Response.code}"></span></li>
<li>응답메시지 (Response.message) : <span th:text="${Response.message}"></span></li>
<li>국세청승인번호 (Response.ntsConfirmNum) : <span th:text="${Response.ntsConfirmNum}"></span></li>
</ul>
</fieldset>
</body>
</html>
③ Call web-browser to check the result.
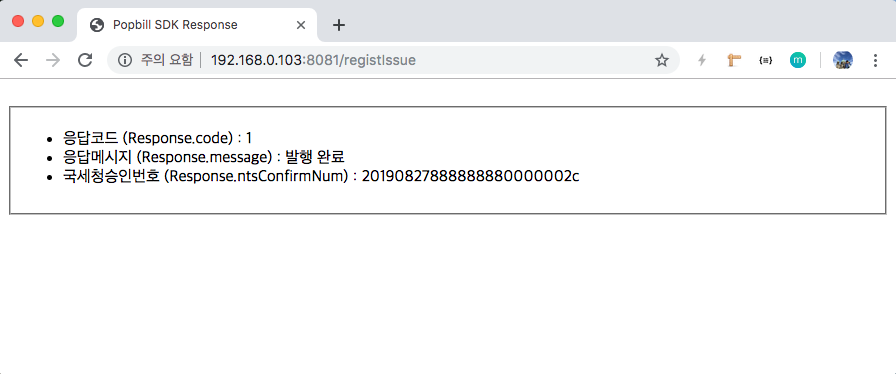
Business Contact
For more help with POPBiLL, try these resources :
T. +82 70-7998-7117E. global@linkhubcorp.com